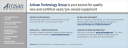
advertisement
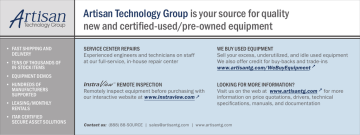
A
VMIVME-2540 Intelligent Counter/Controller
Programming Example
/*** Name: Test2540.c Demonstration Program for VMIVME-2540 board
Description: This software was written to accommodate all the
features of the 2540 product. To accomplish this
objective, the program makes extensive use of
pointers to all the data types and parameters
in the CCB area of the shared memory space
contained on the VMIVME-2540 board and uses
many byte arrays to define data attributes. This
approach allows any channel to be configured for
any operation, with prompt provide to allow the
function selection. This approach would not be
necessary in a given application for the board,
allowing a much simpler program to be used for
dedicated channel operation. All pointers
required for any operation are computed at the
entry of the user-selected channel number, with
the pointers computed as a function of the channel
number for the subsequent operation. The program
uses polling exclusively, and uses delay loop counts
for paced access to the board.
Notes: The program is code for a board base address of 0xFB200000.
To accommodate another address, change BASE_ADDRESS prior to compilation.
***/
#define BASE_ADDRESS 0xFB200000
#define MAXACC 20
#define MAX_MWAIT 2000000000
#define MAX_CMD_RESP 200
#define MIN_DELAY 100 /* minimum delay of 50 usec */
#define NULL 0x00
#define FALSE 0
#define TRUE -1
#define VAL_ID 0x25
#define BRD_OPT 0x03
#define DISABLE_CH 0x00
#define EVENT_16 0x01
#define EVENT_16_GATE 0x02
#define EVENT_32 0x03
#define EVENT_32_GATE 0x04
#define READ_CURR_EVT_CNT 0x06
#define FREQ_DIV_16 0x07
#define FREQ_DIV_32 0x08
#define SQ_WAVE 0x09
#define PULS_TRAIN 0x0A
#define FREQ_GEN 0x0B
120
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
#define DUTY_CYCLE 0x0C
#define MEAS_FLT_PER 0x0D
#define MEAS_FLT_PER_ENH 0x0E
#define MEAS_FLT_FREQ 0x0F
#define MEAS_FLT_FREQ_ENH 0x10
#define MEAS_PULS_WIDTH_FLT 0x11
#define MEAS_PULS_WIDTH_FLT_ENH 0x12
#define MEAS_QUAD_POS_FLT 0x13
#define READ_QUAD_POS_FLT 0x15
#define MEAS_QUAD_POS_INT 0x16
#define READ_QUAD_POS_INT 0x17
#define TIMER_INTERRUPT_SNG 0x18
#define TIMER_INTERRUPT_MULT 0x19
#define DISABLE_TIMER 0x1A
#define INITIALIZE 0x1B
#define RESET_STATUS 0x1C
#define BLOCK_MOVE 0x1D
#define EXECUTE 0x1E
#define ECHO_PC 0x1F
#define MEAS_PER16 0x20
#define PULS_SEQ 0x21
#define PGM_IO 0x22
#define QUAD_POS_CTL 0x23
#define GROUP_ACQ 0x24
#define EVENT_DELAY_TRIG 0x25
#define MEAS_PULS_WIDTH_INT 0x26
#define MEAS_PER32 0x27
#define MEAS_PUL32 0x28
#define ACT_HI 0x00
#define ACT_LO 0x20
#define MEAS_RDY 0xFF
#define TFLAG 0xFF
#define ACK 0x01
#define EVT_RDY 0x02
#define PER_RDY 0x03
#define FRQ_RDY 0x04
#define PUL_RDY 0x05
#define QPM_RDY 0x06
#define LIM_ALARM 0x07
#define INT_32 1
#define INT_16 2
#define FLT_32 3
#define SINT_32 4
#define DUAL_SINT_32 5
#define INPUT 0
#define OUTPUT 0xFF
#define PRE_SCALE 0xFF
#define RESTART -1
#define EXIT -2
#define NUM_INPUTS 18
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
121
A
VMIVME-2540 Intelligent Counter/Controller
#define SNGL 0
#define DUAL 1
/* Define flags for input operation mode */
#define CCB_STAT 0x01
#define MFLAG 0x02
#define MQUEUE 0x04
#define CONTINUOUS 0x80 /* opn_flag: continuous mode indicator */
#define OPN_COMPLETE 0xFF
#define DISCRETE 0x10 /* opn_flag: discrete mode */
#define SET_UP 0x08 /* opn_flag: setup only */
/* Define the configuration channel size for each option */ char *nchptr[] = { "4", "8", "16", "24" };
/* Define the command status and CCB status codes indexed by code */ char *diag_msg[] = { "", "Command Acknowledge", "Event count ready",
"Period Measurement ready","Freq. Measurement ready",
"Pul. Wdth. Measurement ready", "Quad. pos. measurement ready",
"Limit Alarm", "Timer Alarm", "Channel Allocation error",
"Bounds error", "Period error", "Pulse Width error",
"Freq. error", "Scale error", "Quad. Pos. meas. error",
"Gate error", "Limit error", "Active channel error",
"Request Denied", "Under-range measurement",
"QPM clockwise overflow", "QPM counter-clockwise overflow" };
/* Define a message timeout error code string */ char timer_str[] = "Measurement flag timeout\r\n";
/* Message for any code not in above list */ char unk_str[] = "Unknown return code\r\n";
/* Define the quadrature messages codes */ char *quad_diag[] = { "", "Clockwise 32-bit Overflow", "Counterclockwise"
" 32-bit overflow", "Clockwise Limit Exceeded",
" Counterclockwise limit exceeded" };
/* Define any fatal/non-fatal attribute for each error code */ char fatal_flg[] = { FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE,
FALSE, TRUE, TRUE, TRUE, TRUE, TRUE, TRUE, TRUE, TRUE,
TRUE, TRUE, TRUE, FALSE }; char chnl_active[24] = { FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE,
FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE,
FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE };
/* define an array of address (1 for each channel) which point to the meas.
location in the CCB. */ unsigned char *chnl_meas_ptr[24] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL };
122
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
/* define an array of addresses (1 per channel) which point to CCB status */ unsigned char *chnl_status_ptr[24] = { NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL };
/* define the commands by program index */ unsigned char cmd_sel[27] = { MEAS_PER32, MEAS_PUL32, MEAS_PER16, MEAS_FLT_PER,
MEAS_FLT_PER_ENH, MEAS_FLT_FREQ, MEAS_FLT_FREQ_ENH, EVENT_16,
EVENT_32, MEAS_PULS_WIDTH_FLT, MEAS_PULS_WIDTH_FLT_ENH,
MEAS_QUAD_POS_FLT, MEAS_QUAD_POS_INT, GROUP_ACQ,READ_CURR_EVT_CNT,
READ_QUAD_POS_FLT,READ_QUAD_POS_INT, EVENT_DELAY_TRIG, SQ_WAVE,
PULS_TRAIN, FREQ_GEN, DUTY_CYCLE, PULS_SEQ, FREQ_DIV_16,
FREQ_DIV_32, PGM_IO, QUAD_POS_CTL }; unsigned char cmd_index_tbl[41] = { FALSE, 7, 7, 8, 8, FALSE, 14, FALSE,
FALSE, FALSE, FALSE, FALSE, FALSE, 3, 4, 5, 6, 9, 10, 11, FALSE,
FALSE, 12, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE, FALSE,
FALSE, 2, FALSE, FALSE, FALSE, 13, 17, FALSE, 0, 1 };
/* define the data types for screen display */ char opn_data_types[27] = { INT_32, INT_32, INT_16, FLT_32, FLT_32, FLT_32,
FLT_32, INT_16, INT_32, FLT_32, FLT_32, FLT_32,
SINT_32, DUAL_SINT_32, FLT_32, FLT_32, SINT_32,
FLT_32, FLT_32, FLT_32, FLT_32, FLT_32, FLT_32,
FLT_32, FLT_32, FLT_32, SINT_32 };
/* flag the command types needing a pre-scalar */ char prescl_flg[27] = { NULL, NULL, NULL, NULL, PRE_SCALE, NULL, PRE_SCALE,
NULL, NULL, NULL, PRE_SCALE, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL };
/* flag the command types that use period measurement floating point */ char flt_per_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, FLT_32, FLT_32, NULL, NULL, NULL, NULL,
NULL, NULL, NULL };
/* flag the command types that use frequency measurement floating point */ char flt_frq_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, FLT_32, FLT_32, NULL, NULL,
NULL, NULL, NULL };
/* flag the command types that use pulse width measurement floating point */ char flt_pw_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, FLT_32, NULL, NULL, NULL, NULL,
NULL, NULL, NULL };
/* flag the command types that use 16-bit and 32-bit frequency division */ char freq_div_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
123
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, INT_16,
INT_32, NULL, NULL };
/* flag the command types that use gate input signals */ char gate_flg[27] = { NULL, TFLAG, NULL, NULL, NULL, NULL, NULL, TFLAG,
TFLAG, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL };
/* flag the command types that use clock selects */ char clock_flg[27] = { TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, NULL,
NULL, TFLAG, TFLAG, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, TFLAG, NULL, NULL,
NULL, TFLAG };
/* flag the command types that use limit parameters */ char lim_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, TFLAG,
TFLAG, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL };
/* flag the command types that use a duty_cycle parameter */ char duty_cyc_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, TFLAG, NULL, NULL, NULL, NULL,
NULL };
/* flag the command types that use clocks counts high and low for signal def */ char clock_sel_flg[27] = { NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, TFLAG, NULL, NULL, NULL,
NULL };
/* flag the command types that use sample sizes */ char sampl_flg[27] = { NULL, NULL, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, NULL,
NULL, TFLAG, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL };
/* flag the command types where continuous/discrete is applicable */ char cont_flg[27] = { TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG,
TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG, TFLAG,
TFLAG, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
NULL, NULL };
/* define the measure status expected for normal measurement completion */ unsigned char stat_sel[27] = { PER_RDY, PUL_RDY, PER_RDY, PER_RDY, PER_RDY,
FRQ_RDY, FRQ_RDY, EVT_RDY, EVT_RDY, PUL_RDY,
PUL_RDY, QPM_RDY, QPM_RDY, QPM_RDY, EVT_RDY,
QPM_RDY, QPM_RDY, LIM_ALARM, NULL, NULL, NULL,
NULL, NULL, NULL, NULL, OPN_COMPLETE };
/* Define all prompt message strings */ char sel_period[] = "\r\nSelect the period desired (Sec. - xxx.yy): >"; char sel_freq[] = "\r\nSelect the frequency desired (Hz. - xxx.yy): >";
124
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A char sel_puls_width[] = "\r\nSelect the pulse width desired (Sec. - xxx.yy): >"; char sel_duty_cycle[] = "\r\nSelect the duty cycle desired (\% - xxx.yy): >"; char sel_high_clocks[] = "\r\nSelect high level duration"
" (number of clocks): >"; char sel_low_clocks[] = "\r\nSelect low level duration"
" (number of clocks): >"; char sel_total_dur[] = "\r\nSelect total pulse sequence duration (2-32767) : >"; char div_prm[] = "\r\nEnter Divisor: >"; char opn_type[] = "\r\n\nSelect operation type\r\n"
" (0 = INPUT; 1 = OUTPUT; 2 = DISABLE): >"; char meas_type[] = "\r\n\nSelect Measurement Type\r\n"
" 0 = 32-bit Integer Period 1 = 32-bit Integer Pulse Width\r\n"
" 2 = 16-bit Integer Period
" 4 = Enhanced Floating Period
3 = Floating Period\r\n"
5 = Floating Frequency\r\n"
" 6 = Enhanced Floating Frequency 7 = 16-bit Integer Event Count\r\n"
" 8 = 32-bit Integer Event Count 9 = Floating Pulse Width\r\n"
" 10 = Enhanced Floating Pulse
" 12 = Quad Pos. Integer Measuremnt
11 = Quad Pos. Floating Measurement\r\n"
13 = Quad Group Acquisition\r\n"
" 14 = Read Current Event Count 15 = Read Quad Position Float\r\n"
" 16 = Read Quad Position Int.
">";
17 = Event Delay Trigger\r\n" char output_type[] = "\r\nSelect Output Operation\r\n"
" 0 = Square Wave (50/50) 1 = Pulse Train\r\n"
" 2 = Frequency (50/50) 3 = Duty Cycle\r\n"
" 4 = Pulse Sequence 5 = 16 bit Frequency Divider\r\n"
" 6 = 32-bit Frequency Divider 7 = Programmed Output\r\n"
" 8 = Quadrature Position Control\r\n"
">"; char qpc_pw_sel[] = "\r\nSelect the output pulse width (as the number of\r\n"
"clock source cycles): >"; char qpc_delta_sel[] = "\r\nSelect the delta position (in output pulses): >"; char qpc_pos_sel[] = "\r\nSelect the current position location (in output\r\n"
" cycles): >"; char wait_outmsg[] = "\r\nWait for the angle change to complete (y or n): >"; char opn_prm[] = "\r\n\nSelect input operation mode\r\n"
"(0 = single; 1 = continuous; 2 = setup only; "
"3 continuous-setup): >"; char chnnum[] = "\r\n\nEnter Channel number: >"; char sel_pulse[] = "\r\nSelect active gate level/active clock edge\r\n"
"(0 = active high gate/rising clock; 1 = active high gate/falling clock;\r\n"
" 2 = active low gate/rising clock; 3 = active high gate/falling clock):\r\n"
" 4 = no active gating: >"; char sel_clock[] = "\r\n"
"Select clock frequency desired (0 = autoranging; 1 = 200 nsec.;\r\n"
"2 = 2 usec.; 3 = 20 usec; 4 = 200 usec.; 5 = 2 msec.): >"; char sel_limit[] = "\r\nSelect limit for the limit alarm: >"; char sel_level[] = "\r\nSelect output level (0 = low; 1 = high): >"; char mode_prm[] = "\r\n\nEnter mode (0 = report every measurement;"
" 1 = average \"N\" measurement;"
"\r\n 2 = discard \"N\" measurements) : >";
125
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
char sampl_prm[] = "\r\nEnter sample size (0-65535): >"; char prescale[] = "\r\nEnter a prescaler choice (0-65535): >\r\n"; char pang_lim_sel[] = "\r\nEnter clockwise angle limit: >"; char nang_lim_sel[] = "\r\nEnter counter-clockwise angle limit: >"; char scale_sel[] = "\r\nEnter a scale facter (degrees/pulse): >"; char sel_clock_edge[] = "\r\nSelect the desired input edge\r\n"
"(0 = falling; 1 = rising): >"; char spres_flag[] = "\r\nRetriggering suppressed (y or n)? >"; char sel_delay_time[] = "\r\nEnter the desired delay from the input edge\r\n"
"in seconds (xx.yyyyy): >"; char status_poll[] = "\r\n"
"Poll the CCB status (1), Measure Ready flag (2) or\r\n"
"Measurement Queue flag (3): >"; char display_meas[] = "\r\n"
"Display the measurement data (y/n)"; char restart_opt[] = "\r\nAutomatic restart (y or n): >"; char cont_meas[] = "\r\nContinue operations on active channel (y or n)? >"; char operation_sel[] = "\r\nEnter:\r\n"
"\"r\" to disable;\r\n"
"\"a\" to select another channel with current channel active;\r\n"
"\"c\" to continue with current channel measurements;\r\n"
"\"x\" to exit;\r\n"
"any other key for disable/enable restart sequence...\r\n"
"> "; char continue_msg[] = "\r\nEnter \"y\" to continue from current position,"
"\"n\" to re-initialize: >"; char anykey[] = "\r\nPress any key to continue\r\n"; char invalid_sel[] = "\r\nInvalid selection - Re-enter\r\n"; char inv_lvl[] = "\r\nInvalid entry for this operation - Re-enter\r\n"; char inv_brd_id[] = "\r\nBoard installed has incorrect ID code.\r\n"; struct vmivme_2540_regs {
unsigned short bid; unsigned short rev; unsigned short cmd;
unsigned char unused; unsigned char response; unsigned char resp_irq;
unsigned char resp_vec; unsigned char channel; unsigned char contdisc;
unsigned short mflag; unsigned char mchan; unsigned char mcode;
struct CH_CB {
unsigned char command; unsigned char gate_edge; unsigned char vme_irq;
unsigned char vme_vec;
union {
unsigned char cp_b[12]; unsigned short cp_w[6]; unsigned int cp_l[3];
float cp_f[3];
} cp;
} ch_ccb[24];
struct TMR_CB {
unsigned char tcmd; unsigned char tgate; unsigned char vme_irq;
unsigned char vme_vec;
union {
unsigned char tp_ub[12]; char tp_b[12]; unsigned short tp_uw[6];
short tp_w[6]; unsigned int tp_ul[3]; int tp_l[3];
126
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
float tp_f[3];
} tp;
} tmr_ccb[6];
unsigned char cd[24]; unsigned char valid_flgs[24];
unsigned char reserv[480]; unsigned short diag_bufr[32];
} *brd_ptr = (struct vmivme_2540_regs *)BASE_ADDRESS; char wait_for_resp(), wait_for_meas(), poll_resp_code(); int prompti(); float promptf(); void wait_for_valid(), print_meas(), access_delay(); short ncounts; static int ccb_length = 16; unsigned char meas_st, *meas_addr, poll_flag, active_cmd, meas_cmd; unsigned char *mstatus, *cvalue, *valid_flg, *brd_id_ptr,
*clk_value, *con_disc, *curr_cmd, *curr_op_mode; unsigned char clock_sel, data_typ, data_dir, chnl_cmd, read_opn, clk_edge,
restart, opn_flag, sflag; char dis_flag; unsigned short *diagnostics, *command, *svalue, *freq_div;
/* Allocate pointers to access the various parameter types */ unsigned char *uc_ptr0, *uc_ptr1, *uc_ptr2, *uc_ptr4, *uc_ptr5,
*uc_ptr8, *uc_ptr10; short *s_ptr0, *s_ptr1; unsigned short *us_ptr0, *us_ptr1, *us_ptr2, *us_ptr5; unsigned int *ul_ptr0, *ul_ptr1; float *flt_ptr0, *flt_ptr1, *flt_ptr2;
/* allocate variables for entry by user */ short qpc_delta; unsigned short qpc_puls_cnt; int qpc_position, *slvalue, *slvalue1; float freq_sel, puls_sel, pos_ang_lim, neg_ang_lim, ang_scale, tdelay;
/* Allocate pointers to selected channels */ struct CH_CB *brd_chnl, *brd_chnl1; void main()
{
static char opt_chnls[4] = { 4, 8, 16, 24 };
int ntime, cmd_index, act_pulse, act_chnl, in_samp, op_type,
op_mode, pscale_clk, max_chnls;
unsigned char brd_id, out_level, m_mode, res_flag;
127
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
short cmd_wait, i;
unsigned short clk_low, clk_high, clk_dur;
unsigned long f_divisor, limit;
char in_char, ch_opt, div_flag, cmd_status, wait_out, quad_con;
unsigned int *ev_32_lim_ptr;
command = (unsigned short *)&brd_ptr->cmd;
diagnostics = (unsigned short *)&brd_ptr->diag_bufr[0]; begin:
/* Display board menu */ sel_chn:
res_flag = FALSE;
act_chnl = prompti(chnnum); /* prompt for channel number */
if (act_chnl == EXIT)return;
/* Now access the board, retrieving the ID and option and allow enough
time for board to complete initialization (if it was reset/power-on). */
brd_id_ptr = (unsigned char *)&brd_ptr->bid;
while (*brd_id_ptr != VAL_ID) {
ntime--;
access_delay();
if (ntime <= 0) {
fast_print(inv_brd_id);
return; }
}
con_disc = &brd_ptr->contdisc; /* set continuous discrete flag ptr */
brd_ptr->channel = act_chnl; /* set pointer to selected channel */
ch_opt = *(brd_id_ptr+1); /* retrieve board option */
max_chnls = (int )opt_chnls[ch_opt]; /* retrieve number of channels */
printf("\r\nNumber of channels = %2d", max_chnls);
if (act_chnl > max_chnls) { /* check selected channel */
fast_print(invalid_sel);
goto sel_chn; }
/* First send the reset command status code */
*command = (unsigned short )RESET_STATUS;
if (wait_for_resp())goto sel_chn;
/* set the active channel number in the header shared memory space */
brd_ptr->channel = act_chnl;
/* set default non-read operation flag */
read_opn = FALSE;
/* set default zero length pulse width */
act_pulse = 0;
valid_flg = &brd_ptr->valid_flgs[act_chnl];
brd_chnl = &brd_ptr->ch_ccb[act_chnl];
128
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
brd_chnl1 = &brd_ptr->ch_ccb[act_chnl+1];
curr_cmd = (unsigned char *)&brd_chnl->command;
cvalue = (unsigned char *)&brd_chnl->gate_edge;
uc_ptr0 = &brd_chnl->cp.cp_b[0];
uc_ptr1 = &brd_chnl->cp.cp_b[1];
uc_ptr2 = &brd_chnl->cp.cp_b[2];
uc_ptr4 = &brd_chnl->cp.cp_b[4];
uc_ptr5 = &brd_chnl->cp.cp_b[5];
uc_ptr8 = &brd_chnl->cp.cp_b[8];
uc_ptr10 = &brd_chnl->cp.cp_b[10];
flt_ptr0 = (float *)uc_ptr0;
flt_ptr1 = (float *)uc_ptr4;
flt_ptr2 = (float *)uc_ptr8;
ul_ptr0 = (unsigned int *)uc_ptr0;
ul_ptr1 = (unsigned int *)uc_ptr4;
us_ptr0 = (unsigned short *)uc_ptr0;
us_ptr1 = (unsigned short *)uc_ptr2;
us_ptr2 = (unsigned short *)uc_ptr4;
us_ptr5 = (unsigned short *)uc_ptr10;
s_ptr0 = (short *)us_ptr0;
s_ptr1 = (short *)us_ptr1;
clk_value = uc_ptr1;
meas_addr = uc_ptr4;
slvalue = (int *)uc_ptr4;
svalue = us_ptr1;
active_cmd = *curr_cmd;
curr_op_mode = &brd_ptr->cd[act_chnl];
if (active_cmd != 0)
if (prompt(cont_meas) == ’y’) {
meas_cmd = active_cmd;
cmd_index = cmd_index_tbl[active_cmd];
if (cmd_index == FALSE) {
printf("\r\nCan’t continue this channel.\r\n");
res_flag = FALSE;
goto sel_chn; }
res_flag = TRUE;
opn_flag = chnl_active[act_chnl];
data_typ = opn_data_types[cmd_index];
mstatus = chnl_status_ptr[act_chnl];
meas_st = stat_sel[cmd_index];
meas_addr = chnl_meas_ptr[act_chnl];
data_dir = INPUT;
op_type = INPUT;
if (opn_flag & CONTINUOUS)goto cloop;
else goto resume_m; /* discrete channel operations */
} sel_opn:
op_type = prompti(opn_type); /* select operation type */
if (op_type == EXIT)return;
if (op_type == RESTART)goto sel_chn;
Programming Example
A
129
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
if (op_type == 2) {
*command = (unsigned short )DISABLE_CH;
wait_for_resp();
goto sel_chn;
}
if (op_type == 0) {
data_dir = INPUT;
cmd_index = prompti(meas_type);
}
else
if (op_type == 1) {
data_dir = OUTPUT;
opn_flag = DISCRETE;
cmd_index = prompti(output_type);
cmd_index = cmd_index + NUM_INPUTS;
}
else {
fast_print(invalid_sel);
goto sel_opn;
}
div_flag = freq_div_flg[cmd_index];
if (cmd_index == EXIT)return;
/* Continue to process menu selection */
data_typ = opn_data_types[cmd_index];
meas_cmd = cmd_sel[cmd_index];
meas_st = stat_sel[cmd_index];
mstatus = uc_ptr8;
if (meas_cmd == MEAS_QUAD_POS_FLT) {
pos_ang_lim = promptf(pang_lim_sel);
neg_ang_lim = promptf(nang_lim_sel);
ang_scale = promptf(scale_sel);
meas_addr = uc_ptr0;
}
if (meas_cmd == EVENT_DELAY_TRIG) {
clk_edge = prompti(sel_clock_edge);
tdelay = promptf(sel_delay_time);
sflag = prompt(spres_flag);
if (sflag == ’y’)
sflag = 0xFF;
else sflag = 0;
if (prompt(restart_opt) == ’y’) restart = TRUE;
else restart = FALSE;
}
if (clock_flg[cmd_index]) { re_clk:
clock_sel = (unsigned char)prompti(sel_clock);
if ((clock_sel < 0) || (clock_sel > 5)) {
fast_print(invalid_sel);
goto re_clk; }
130
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
}
if (prescl_flg[cmd_index] == (char )PRE_SCALE)
pscale_clk = prompti(prescale);
else pscale_clk = 0;
if (gate_flg[cmd_index]) {
act_pulse = prompti(sel_pulse); /* prompt for act. low/high */
if (act_pulse != 4) {
if (meas_cmd < READ_CURR_EVT_CNT) meas_cmd += 1; }
else
act_pulse = 0;
}
if (lim_flg[cmd_index]) { re_lim: limit = prompti(sel_limit);
if (meas_cmd < EVENT_32)
if (limit > 65535) {
fast_print(inv_lvl);
goto re_lim; }
}
if (cmd_index < 2) { re_m_mode:
m_mode = (unsigned char )prompti(mode_prm);
if (m_mode > 2) {
fast_print(invalid_sel);
goto re_m_mode; }
if (m_mode != 0) sampl_flg[cmd_index] = TFLAG;
else sampl_flg[cmd_index] = NULL;
}
if (sampl_flg[cmd_index])
in_samp = prompti(sampl_prm);
if (flt_per_flg[cmd_index])
freq_sel = promptf(sel_period);
if (flt_frq_flg[cmd_index])
freq_sel = promptf(sel_freq);
if (flt_pw_flg[cmd_index])
puls_sel = promptf(sel_puls_width);
if (duty_cyc_flg[cmd_index])
puls_sel = promptf(sel_duty_cycle);
if (clock_sel_flg[cmd_index]) {
clk_low = prompti(sel_low_clocks);
clk_high = prompti(sel_high_clocks);
clk_dur = prompti(sel_total_dur);
}
if (div_flag) { re_div: f_divisor = prompti(div_prm);
if (f_divisor < 2) goto div_err;
else
if (f_divisor > 65535)
if (div_flag == INT_16) { div_err: fast_print(inv_lvl);
goto re_div; }
131
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
}
if (meas_cmd == PGM_IO) { re_lvl: out_level = (unsigned char )prompti(sel_level);
if ((out_level < 0) || (out_level > 1)) {
fast_print(invalid_sel);
goto re_lvl; }
}
else
if (meas_cmd == MEAS_PER16)
mstatus = uc_ptr2;
else
if (meas_cmd == QUAD_POS_CTL) { qloop:
qpc_delta = (short )prompti(qpc_delta_sel);
if (*curr_cmd == QUAD_POS_CTL)
if (prompt(continue_msg) == ’y’) {
quad_con = TRUE;
goto del_only;
}
quad_con = FALSE;
qpc_puls_cnt = (unsigned short )prompti(qpc_pw_sel);
qpc_position = (int )prompti(qpc_pos_sel); del_only:
printf("\r\ndelta angle = %d", qpc_delta);
wait_out = prompt(wait_outmsg);
mstatus = uc_ptr10;
} resume_m:
if (cont_flg[cmd_index]) {
op_mode = prompti(opn_prm); /* prompt for discrete/continuous */
if (op_mode == 0) opn_flag = DISCRETE;
else if (op_mode == 1)opn_flag = CONTINUOUS;
else if (op_mode == 2)opn_flag = SET_UP;
else opn_flag = CONTINUOUS | SET_UP;
}
if (data_dir == INPUT) {
poll_flag = (unsigned char)prompti(status_poll);
dis_flag = prompt(display_meas);
if (dis_flag != ’y’)dis_flag = NULL;
}
poll_flag &= 0x03;
if (poll_flag == 0x03)poll_flag++;
if (opn_flag & CONTINUOUS) opn_flag |= poll_flag;
chnl_active[act_chnl] = opn_flag;
/* Set pointers to diagnostic buffer and board and
clear the diagnostics buffer */
diagnostics = (unsigned short *)&brd_ptr->diag_bufr[0];
for (i = 0; i < 12; i++) *diagnostics++ = 0;
132
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
ntime = MAXACC;
*command = RESET_STATUS;
if (wait_for_resp()) goto sel_chn;
in_char = NULL; mloop:
if (res_flag) {
res_flag = FALSE;
goto send_cmd;
}
if (meas_cmd < FREQ_DIV_16) {
*cvalue = act_pulse;
if (meas_cmd < EVENT_32) {
meas_addr = (unsigned char *)uc_ptr2;
*us_ptr0 = (unsigned short)limit;
}
else
if (meas_cmd < READ_CURR_EVT_CNT) {
if (clock_sel == 4) clock_sel = 0;
*ul_ptr0 = limit;
}
else { /* Read current event count */
read_opn = TRUE;
chnl_cmd = *curr_cmd;
if (chnl_cmd < EVENT_32)
data_typ = INT_16;
else
data_typ = INT_32;
goto send_cmd; /* command is a read event count */
}
}
else
if (meas_cmd < FREQ_DIV_32)
*us_ptr0 = (unsigned short )f_divisor;
else
if (meas_cmd < SQ_WAVE)
*ul_ptr0 = f_divisor;
else
if ((meas_cmd < MEAS_FLT_PER)) {
*flt_ptr0 = freq_sel;
*flt_ptr1 = puls_sel;
}
else
if (meas_cmd < MEAS_QUAD_POS_FLT) {
*cvalue = (unsigned char )clock_sel;
*us_ptr5 = (unsigned short )pscale_clk;
*us_ptr0 = (unsigned short )in_samp;
}
else
if (meas_cmd < MEAS_QUAD_POS_INT) {
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
133
A
VMIVME-2540 Intelligent Counter/Controller
mstatus = cvalue;
if (meas_cmd == READ_QUAD_POS_FLT) {
read_opn = TRUE;
goto send_cmd; }
else {
*flt_ptr0 = ang_scale;
*flt_ptr1 = pos_ang_lim;
*flt_ptr2 = neg_ang_lim;
}
}
else
if (meas_cmd < TIMER_INTERRUPT_SNG) {
mstatus = cvalue;
meas_addr = uc_ptr0;
if (meas_cmd == READ_QUAD_POS_INT) read_opn = TRUE;
}
else
if (meas_cmd < PULS_SEQ) {
*cvalue = (unsigned char )clock_sel;
meas_addr = uc_ptr0;
}
else
if (meas_cmd < PGM_IO) {
*cvalue = (unsigned char)clock_sel;
*us_ptr0 = clk_low;
*us_ptr1 = clk_high;
*us_ptr2 = clk_dur;
}
else
if (meas_cmd < QUAD_POS_CTL)
*cvalue = out_level;
else
if (meas_cmd < GROUP_ACQ) {
*cvalue = (unsigned char)clock_sel;
*s_ptr1 = qpc_delta;
if (quad_con) goto send_cmd;
else {
*ul_ptr1 = qpc_position;
*us_ptr0 = qpc_puls_cnt;
}
}
else
if (meas_cmd < EVENT_DELAY_TRIG) {
mstatus = cvalue;
slvalue = (int *)ul_ptr0;
slvalue1 = (int *)((int )slvalue + (2 * ccb_length));
read_opn = TRUE;
}
else
if (meas_cmd < MEAS_PULS_WIDTH_INT) {
134
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
*cvalue = clk_edge;
*flt_ptr0 = tdelay;
*uc_ptr4 = restart;
*uc_ptr5 = sflag;
}
else
if (meas_cmd > MEAS_PULS_WIDTH_INT) {
*cvalue = act_pulse; /* set the active pulse level */
*uc_ptr0 = m_mode; /* set msnmnt mode */
*clk_value = clock_sel; /* select the active clock */
*us_ptr1 = (unsigned short )in_samp; /* set sample size */
}
else
*cvalue = 0; send_cmd:
chnl_meas_ptr[act_chnl] = meas_addr;
chnl_status_ptr[act_chnl] = mstatus;
if (opn_flag & CONTINUOUS)
*con_disc = 0xff;
else
*con_disc = 0;
*valid_flg = 0;
*mstatus = 0; /* clear status */
*command = meas_cmd;
if (wait_for_resp())goto sel_chn;
if (data_dir == OUTPUT) {
if (meas_cmd != QUAD_POS_CTL)
goto sel_chn;
else
if (wait_out == ’n’)goto sel_chn;
} cloop:
if (op_type == 0)
if (opn_flag & SET_UP) {
printf("\r\nInput setup complete\r\n");
chnl_active[act_chnl] = opn_flag ^ SET_UP;
goto sel_chn;
}
if (opn_flag & 0x01);
else
if (opn_flag & 0x02) {
mstatus = valid_flg;
meas_st = 0xff;
}
else
if (opn_flag & 0x04) {
mstatus = (unsigned char *)&brd_ptr->mflag;
meas_st = 0xff;
}
if (meas_st == NULL) goto begin;
Programming Example
A
135
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
else {
if (opn_flag & CONTINUOUS)
while (!kbhit()) {
cmd_status = wait_for_meas();
if (cmd_status) break;
if (read_opn) *command = meas_cmd;
}
else wait_for_meas();
brd_ptr->channel = act_chnl;
/* Channel disable - send selected command */
in_char = prompt(operation_sel);
if (in_char == ’x’)return;
else
if (in_char == ’c’)
if (*curr_cmd == QUAD_POS_CTL)goto qloop;
else if (data_dir == INPUT)goto cloop;
else goto sel_chn;
else
if (in_char == ’a’) goto sel_chn;
*command = (unsigned short )DISABLE_CH;
if (wait_for_resp())goto sel_chn;
if (in_char == ’r’)goto begin;
if (read_opn)goto sel_chn;
else goto mloop;
}
}
/* Define the function which waits for a command status and processes
the status received from the polling routine. */ char wait_for_resp(void)
{
short cmd_wait, i, j;
char rtn_code, cmd_out;
cmd_wait = 0;
rtn_code = 0;
cmd_out = *command;
while (rtn_code == 0) {
rtn_code = poll_resp_code();
if (cmd_out == RESET_STATUS)
if (rtn_code == 0) return(FALSE);
else rtn_code = 0;
cmd_wait++;
if (cmd_wait > MAX_CMD_RESP) {
printf("\r\n***No response for command***\r\n");
return(TRUE);
}
}
136
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
if (*diagnostics != 0) {
printf("\r\nCommand fatal error - diagnostic buffer:\r\n");
for (i = 0; i < 7; i++) {
for (j = 0; j < 6; j++)
printf("0x%04x ",*diagnostics++);
printf("\r\n");
}
}
if (read_opn)
if (rtn_code == meas_st) return (FALSE);
if (rtn_code != ACK) {
printf("\r\nCommand error code = %02x: %s\r\n", rtn_code,
diag_msg[rtn_code]);
return (fatal_flg[rtn_code]);
}
if (cmd_out == DISABLE_CH)
printf("\r\nChannel disable acknowledged\r\n");
else
if (data_dir == OUTPUT)
printf("\r\nOutput setup complete\r\n");
return (FALSE);
} void wait_for_valid()
{
int vdelay;
vdelay = MAX_CMD_RESP;
while (~*valid_flg) {
access_delay();
vdelay--;
if (vdelay <= 0) {
printf("\r\nData Valid Flag Timeout\r\n");
return; }
}
*valid_flg = 0;
if (dis_flag)print_meas();
return;
}
/* Define a function to access the CCB status waiting for a measurement and
using a short delay between accesses (50 usec 68030 @ 25 MHz)*/ char wait_for_meas()
{
unsigned int mdelay;
unsigned char astatus;
char meas_rtn, *diag_str;
mdelay = MAX_MWAIT;
137
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
if (astatus == 0xff)
printf("\r\nWaiting for qpc angle delta completion\n");
meas_rtn = FALSE;
while (mdelay) {
mdelay--;
if (mdelay <= 0)break;
access_delay();
astatus = *mstatus;
if (astatus != 0) {
*mstatus = 0;
if (astatus == meas_st) {
*valid_flg = 0;
if (dis_flag) print_meas();
}
else {
if (meas_st == 0xff) diag_str = timer_str;
else if (astatus > 22)diag_str = unk_str;
else diag_str = diag_msg[astatus];
if (meas_cmd == MEAS_QUAD_POS_FLT)
diag_str = quad_diag[astatus];
printf("\r\nError status: %02x - %s", astatus,
diag_str);
}
break;
}
}
if (meas_st == 0xff) meas_rtn = FALSE;
else meas_rtn = fatal_flg[astatus];
if (mdelay <= 0) {
printf("\r\nNo response from measurement command\r\n");
meas_rtn = TRUE; }
return(meas_rtn);
}
/* Define a function which waits for a non_zero command status with a short
spin delay between access (~50 usec for the program development environ.) */ char poll_resp_code(void)
{
unsigned char p_resp;
unsigned char *r_resp;
int acc_att;
r_resp = &brd_ptr->response;
p_resp = *r_resp;
acc_att = 0;
while (acc_att < MAX_CMD_RESP) {
acc_att++;
ncounts = MIN_DELAY;
while (--ncounts);
138
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
Programming Example
A
if (*r_resp != p_resp)
p_resp = *r_resp;
else
return (char )p_resp; }
exit();
}
/* Define a spin loop access delay function */ void access_delay(void)
{
ncounts = MIN_DELAY;
while (--ncounts);
}
/* define a function to print any measurement base on its data type;
unsigned short, unsigned long, long, and float. */ void print_meas(void)
{
printf("\r\nmeasurement = ");
if (data_typ == INT_16) printf("%d", *(unsigned short *)meas_addr);
else if (data_typ == INT_32) printf("%lu", *(int *)meas_addr);
else if (data_typ == SINT_32) printf("%ld", *(unsigned int *)meas_addr);
else if (data_typ == FLT_32) printf("%f", *(float *)meas_addr);
else printf("%ld ang1 %ld ang2", *slvalue, *slvalue1);
*valid_flg = 0;
} char scr_buf[80]; int str_rtn, prt_rtn;
/* Define a function to prompt for an long integer value */ int prompti(char *prm_str)
{
unsigned int i_value;
char exit_chr; re_prmi:
fast_print(prm_str);
gets(scr_buf);
exit_chr = scr_buf[0] | 0x20;
/* Test for the "x" and "r" characters to support exit and restart returns */
if (exit_chr == ’x’) return (EXIT);
if (exit_chr == ’r’) return (RESTART);
prt_rtn = sscanf(scr_buf, "%ld", &i_value);
if (prt_rtn != 1)
goto re_prmi;
139
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
A
VMIVME-2540 Intelligent Counter/Controller
return(i_value);
}
/* Define a function to prompt for a floating pt. input value */ float promptf(char *prm_str)
{
float f_value; re_prmf:
fast_print(prm_str);
gets(scr_buf);
prt_rtn = sscanf(scr_buf, "%f", &f_value);
if (prt_rtn == -1)
goto re_prmf;
return(f_value);
}
140
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com
• FAST SHIPPING AND
DELIVERY
• TENS OF THOUSANDS OF
IN-STOCK ITEMS
• EQUIPMENT DEMOS
• HUNDREDS OF
MANUFACTURERS
SUPPORTED
• LEASING/MONTHLY
RENTALS
• ITAR CERTIFIED
SECURE ASSET SOLUTIONS
Artisan Technology Group is your source for quality new and certified-used/pre-owned equipment
SERVICE CENTER REPAIRS
Experienced engineers and technicians on staff at our full-service, in-house repair center
Instra
View
SM
REMOTE INSPECTION
Remotely inspect equipment before purchasing with our interactive website at
www.instraview.com
WE BUY USED EQUIPMENT
Sell your excess, underutilized, and idle used equipment
We also offer credit for buy-backs and trade-ins
www.artisantg.com/WeBuyEquipment
LOOKING FOR MORE INFORMATION?
Visit us on the web at
www.artisantg.com
for more information on price quotations, drivers, technical specifications, manuals, and documentation
Contact us:
(888) 88-SOURCE | [email protected] | www.artisantg.com
advertisement
* Your assessment is very important for improving the workof artificial intelligence, which forms the content of this project
Related manuals
advertisement
Table of contents
- 10 List of Figures
- 12 List of Tables
- 14 Overview
- 15 Disclaimer: Notice About Equivalent Parts
- 16 Reference Material List
- 16 Physical Description and Specifications
- 17 Safety Summary
- 17 Ground the System
- 17 Do Not Operate in an Explosive Atmosphere
- 17 Keep Away from Live Circuits
- 17 Do Not Service or Adjust Alone
- 17 Do Not Substitute Parts or Modify System
- 17 Dangerous Procedure Warnings
- 18 Safety Symbols Used in This Manual
- 20 Chapter 1 - Theory of Operation
- 20 Functional Description
- 20 System Timing Controller Front-End Logic
- 20 RS-422 Line Driver and Receiver
- 21 Synchronizer and Conditioning Logic
- 23 AM9513A System Timing Controller
- 24 QPM Direction Change Interrupt Logic
- 25 I/O Processor
- 25 68HC000 CPU
- 25 Decode and Control Logic
- 25 Local Bus Arbitration
- 27 Local Address Decode
- 29 Local I/O Functions
- 31 Local Memory
- 31 EPROM Firmware
- 31 Static RAM
- 32 Local Interrupt Controller
- 32 STC Interrupts
- 32 VMEbus Command Interrupt
- 34 VMEbus Slave Interface
- 34 VMEbus Slave Address Decode
- 34 Command Status Code
- 36 VMEbus Interrupter Modules
- 38 Chapter 2 - Configuration and Installation
- 39 Unpacking Procedures
- 40 Configuration
- 41 DIP Switch Settings
- 41 Jumper Options
- 43 I/O Connector Pin Assignments
- 46 Recommended Discrete Wire Connectors and Terminal Blocks
- 47 TTL/Single-Ended Input Signal Compatibility Configuration
- 50 Chapter 3 - Programming
- 52 VMEbus Interface Memory Map
- 53 Board ID/Configuration Buffer
- 54 Firmware Revision Level
- 54 Command Code
- 54 Command Status Code
- 55 Command Status Interrupt Request Level
- 56 Command Status Interrupt Vector
- 56 Channel ID
- 56 Continuous/Discrete Flag
- 57 Measurement Ready Flag
- 57 Measurement Channel ID
- 57 Channel Measurement Status
- 58 Channel Control Block Registers
- 58 Timer Channel Control Block
- 59 VMIVME-2540 Continuous/Discrete Flag Buffer
- 59 VMIVME-2540 Measurement Data Valid Flags Buffer
- 59 VMIVME-2540 Firmware Release Information
- 60 VMIVME-2540 Daignostic Buffer
- 61 Command Interface
- 62 Programming Using the Command Interface
- 64 Command Status Codes
- 65 Modes of Operation
- 66 Input Modes of Operation
- 67 Output Modes of Operation
- 67 Timing Modes of Operation
- 68 Channel Control Blocks Common Parameters
- 69 Operation Mode Selection Flag
- 70 Operational Mode Select Flag
- 71 Command Descriptions
- 71 Initialization and Synchronization Command Codes
- 72 Channel Input/Measurement Command Codes
- 72 Integer 16-bit Event Counting
- 73 Integer 32-bit Event Counting
- 75 Period Measurement
- 76 Frequency Measurement
- 77 Pulse-Width Measurement
- 79 Quadrature Position Measurement
- 82 Integer Quadrature Position Measurement
- 83 16-bit Integer Period Measurement
- 84 32-bit Integer Period Measurement
- 86 32-bit Integer Pulse-Width Measurement
- 87 Group Acquisition Mode (Integer QPM)
- 88 16-bit Integer Pulse Measurement
- 89 Delayed Event Timer with VMEbus Interrupt
- 90 Programming Strategies for Input Operations
- 91 Continuous Data Acquisition Mode
- 92 Discrete Data Acquisition Mode
- 94 Channel Output/Waveform Generation Command Codes
- 94 16-bit Frequency Divider
- 95 32-bit Frequency Divider
- 96 Period/Pulse-Width Generation
- 97 Frequency/Duty Cycle Generation
- 97 Pulse Sequence Generation
- 98 Programmed Output Mode
- 99 Quadrature Position Control
- 100 Programming Strategies for Output Operations
- 100 Timer Operation Command Codes
- 100 Timer/Periodic Interrupt
- 101 Auxiliary Commands
- 103 Getting Started
- 118 Appendix A - Example Code
- 119 Terminal Output of Program gs.c
- 121 Programming Example