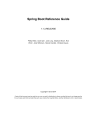
advertisement
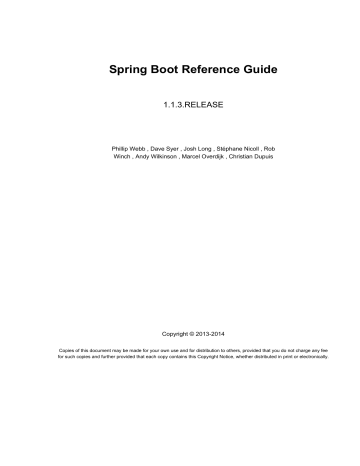
Part VI. Deploying to the cloud
Spring Boot’s executable jars are ready-made for most popular cloud PaaS (platform-as-a-service) providers. These providers tend to require that you `bring your own container'; they manage application processes (not Java applications specifically), so they need some intermediary layer that adapts your application to the cloud’s notion of a running process.
Two popular cloud providers, Heroku and Cloud Foundry, employ a “buildpack” approach. The buildpack wraps your deployed code in whatever is needed to start your application: it might be a JDK and a call to java
, it might be an embedded webserver, or it might be a full fledged application server. A buildpack is pluggable, but ideally you should be able to get by with as few customizations to it as possible. This reduces the footprint of functionality that is not under your control. It minimizes divergence between deployment and production environments.
Ideally, your application, like a Spring Boot executable jar, has everything that it needs to run packaged within it.
In this section we’ll look at what it takes to get the
simple application that we developed
in the “Getting
Started” section up and running in the Cloud.
Spring Boot Reference Guide
44. Cloud Foundry
Cloud Foundry provides default buildpacks that come into play if no other buildpack is specified. The
Cloud Foundry Java buildpack has excellent support for Spring applications, including Spring Boot. You can deploy stand-alone executable jar applications, as well as traditional
.war
packaged applications.
Once you’ve built your application (using, for example, mvn clean package
) and installed the cf command line tool , simply deploy your application using the cf push
command as follows, substituting the path to your compiled
.jar
. Be sure to have logged in with your cf
command line client before pushing an application.
$ cf push acloudyspringtime -p target/demo-0.0.1-SNAPSHOT.jar
See the cf push
documentation for more options. If there is a Cloud Foundry manifest.yml
file present in the same directory, it will be consulted.
Note
Here we are substituting acloudyspringtime
for whatever value you give cf
as the name of your application.
At this point cf
will start uploading your application:
Uploading acloudyspringtime... OK
Preparing to start acloudyspringtime... OK
-----> Downloaded app package (8.9M)
-----> Java Buildpack source: system
-----> Downloading Open JDK 1.7.0_51 from .../x86_64/openjdk-1.7.0_51.tar.gz (1.8s)
Expanding Open JDK to .java-buildpack/open_jdk (1.2s)
-----> Downloading Spring Auto Reconfiguration from 0.8.7 .../auto-reconfiguration-0.8.7.jar (0.1s)
-----> Uploading droplet (44M)
Checking status of app acloudyspringtime...
0 of 1 instances running (1 starting)
...
0 of 1 instances running (1 down)
...
0 of 1 instances running (1 starting)
...
1 of 1 instances running (1 running)
App started
Congratulations! The application is now live!
It’s easy to then verify the status of the deployed application:
$ cf apps
Getting applications in ...
OK name requested state instances memory disk urls
...
acloudyspringtime started 1/1 512M 1G acloudyspringtime.cfapps.io
...
Once Cloud Foundry acknowledges that your application has been deployed, you should be able to hit the application at the URI given, in this case http://acloudyspringtime.cfapps.io/
.
1.1.3.RELEASE
Spring Boot 94
Spring Boot Reference Guide
44.1 Binding to services
By default, meta-data about the running application as well as service connection information is exposed to the application as environment variables (for example:
$VCAP_SERVICES
). This architecture decision is due to Cloud Foundry’s polyglot (any language and platform can be supported as a buildpack) nature; process-scoped environment variables are language agnostic.
Environment variables don’t always make for the easiest API so Spring Boot automatically extracts them and flattens the data into properties that can be accessed through Spring’s
Environment
abstraction:
@Component
class
MyBean
implements
EnvironmentAware {
private
String instanceId;
@Override
public void
setEnvironment(Environment environment) {
this
.instanceId = environment.getProperty(
"vcap.application.instance_id"
);
}
// ...
}
All Cloud Foundry properties are prefixed with vcap
. You can use vcap properties to access application information (such as the public URL of the application) and service information (such as database credentials). See
VcapApplicationListener
Javdoc for complete details.
Tip
The Spring Cloud project is a better fit for tasks such as configuring a DataSource; it also lets you use Spring Cloud with Heroku.
1.1.3.RELEASE
Spring Boot 95
Spring Boot Reference Guide
45. Heroku
Heroku is another popular PaaS platform. To customize Heroku builds, you provide a
Procfile
, which provides the incantation required to deploy an application. Heroku assigns a port
for the Java application to use and then ensures that routing to the external URI works.
You must configure your application to listen on the correct port. Here’s the
Procfile
for our starter
REST application: web: java -Dserver.port=$PORT -jar target/demo-0.0.1-SNAPSHOT.jar
Spring Boot makes
-D
arguments available as properties accessible from a Spring
Environment instance. The server.port
configuration property is fed to the embedded Tomcat or Jetty instance which then uses it when it starts up. The
$PORT
environment variable is assigned to us by the Heroku
PaaS.
Heroku by default will use Java 1.6. This is fine as long as your Maven or Gradle build is set to use the same version (Maven users can use the java.version
property). If you want to use JDK 1.7, create a new file adjacent to your pom.xml
and
Procfile
, called system.properties
. In this file add the following: java.runtime.version=1.7
This should be everything you need. The most common workflow for Heroku deployments is to git push
the code to production.
$ git push heroku master
Initializing repository, done.
Counting objects: 95, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (78/78), done.
Writing objects: 100% (95/95), 8.66 MiB | 606.00 KiB/s, done.
Total 95 (delta 31), reused 0 (delta 0)
-----> Java app detected
-----> Installing OpenJDK 1.7... done
-----> Installing Maven 3.0.3... done
-----> Installing settings.xml... done
-----> executing /app/tmp/cache/.maven/bin/mvn -B
-Duser.home=/tmp/build_0c35a5d2-a067-4abc-a232-14b1fb7a8229
-Dmaven.repo.local=/app/tmp/cache/.m2/repository
-s /app/tmp/cache/.m2/settings.xml -DskipTests=true clean install
[INFO] Scanning for projects...
Downloading: http://repo.spring.io/...
Downloaded: http://repo.spring.io/... (818 B at 1.8 KB/sec)
....
Downloaded: http://s3pository.heroku.com/jvm/... (152 KB at 595.3 KB/sec)
[INFO] Installing /tmp/build_0c35a5d2-a067-4abc-a232-14b1fb7a8229/target/...
[INFO] Installing /tmp/build_0c35a5d2-a067-4abc-a232-14b1fb7a8229/pom.xml ...
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 59.358s
[INFO] Finished at: Fri Mar 07 07:28:25 UTC 2014
[INFO] Final Memory: 20M/493M
[INFO] ------------------------------------------------------------------------
-----> Discovering process types
Procfile declares types -> web
1.1.3.RELEASE
Spring Boot 96
Spring Boot Reference Guide
-----> Compressing... done, 70.4MB
-----> Launching... done, v6
http://agile-sierra-1405.herokuapp.com/ deployed to Heroku
To [email protected]:agile-sierra-1405.git
* [new branch] master -> master
Your application should now be up and running on Heroku.
1.1.3.RELEASE
Spring Boot 97
Spring Boot Reference Guide
46. CloudBees
CloudBees provides cloud-based “continuous integration” and “continuous delivery” services as well as
Java PaaS hosting. Sean Gilligan has contributed an excellent Spring Boot sample application to the
CloudBees community GitHub repository. The project includes an extensive README that covers the steps that you need to follow when deploying to CloudBees.
1.1.3.RELEASE
Spring Boot 98
Spring Boot Reference Guide
47. Openshift
Openshift is the RedHat public (and enterprise) PaaS solution. Like Heroku, it works by running scripts triggered by git commits, so you can script the launching of a Spring Boot application in pretty much any way you like as long as the Java runtime is available (which is a standard feature you can ask for at
Openshift). To do this you can use the DIY Cartridge and hooks in your repository under
.openshift/ action_scripts
:
The basic model is to:
1. Ensure Java and your build tool are installed remotely, e.g. using a pre_build
hook (Java and
Maven are installed by default, Gradle is not)
2. Use a build
hook to build your jar (using Maven or Gradle), e.g.
#!/bin/bash cd $OPENSHIFT_REPO_DIR mvn package -s .openshift/settings.xml -DskipTests=true
3. Add a start
hook that calls java -jar ...
#!/bin/bash cd $OPENSHIFT_REPO_DIR nohup java -jar target/*.jar --server.port=${OPENSHIFT_DIY_PORT} --server.address=${OPENSHIFT_DIY_IP}
&
4. Use a stop
hook (since the start is supposed to return cleanly), e.g.
#!/bin/bash source $OPENSHIFT_CARTRIDGE_SDK_BASH
PID=$(ps -ef | grep java.*\.jar | grep -v grep | awk '{ print $2 }') if [ -z "$PID" ] then
client_result "Application is already stopped" else
kill $PID fi
5. Embed service bindings from environment variables provided by the platform in your application.properties
, e.g.
spring.datasource.url: jdbc:mysql://${OPENSHIFT_MYSQL_DB_HOST}:${OPENSHIFT_MYSQL_DB_PORT}/
${OPENSHIFT_APP_NAME} spring.datasource.username: ${OPENSHIFT_MYSQL_DB_USERNAME} spring.datasource.password: ${OPENSHIFT_MYSQL_DB_PASSWORD}
There’s a blog on running Gradle in Openshift on their website that will get you started with a gradle build to run the app. A bug in Gradle currently prevents you from using Gradle newer than 1.6.
1.1.3.RELEASE
Spring Boot 99
Spring Boot Reference Guide
48. What to read next
Check out the Cloud Foundry , Heroku and CloudBees web sites for more information about the kinds of features that a PaaS can offer. These are just three of the most popular Java PaaS providers, since
Spring Boot is so amenable to cloud-based deployment you’re free to consider other providers as well.
The next section goes on to cover the
; or you can jump ahead to read about
1.1.3.RELEASE
Spring Boot 100
advertisement
* Your assessment is very important for improving the workof artificial intelligence, which forms the content of this project
advertisement
Table of contents
- 1 Spring Boot Reference Guide
- 2 Table of Contents
- 9 Part I. Spring Boot Documentation
- 10 1. About the documentation
- 11 2. Getting help
- 12 3. First steps
- 13 4. Working with Spring Boot
- 14 5. Learning about Spring Boot features
- 15 6. Moving to production
- 16 7. Advanced topics
- 17 Part II. Getting started
- 18 8. Introducing Spring Boot
- 19 9. Installing Spring Boot
- 19 9.1 Installation instructions for the Java developer
- 19 Maven installation
- 20 Gradle installation
- 21 9.2 Installing the Spring Boot CLI
- 21 Manual installation
- 21 Installation with GVM
- 22 OSX Homebrew installation
- 22 Command-line completion
- 22 Quick start Spring CLI example
- 23 9.3 Upgrading from an earlier version of Spring Boot
- 24 10. Developing your first Spring Boot application
- 24 10.1 Creating the POM
- 25 10.2 Adding classpath dependencies
- 25 10.3 Writing the code
- 26 The @RestController and @RequestMapping annotations
- 26 The @EnableAutoConfiguration annotation
- 26 The “main” method
- 26 10.4 Running the example
- 27 10.5 Creating an executable jar
- 29 11. What to read next
- 30 Part III. Using Spring Boot
- 31 12. Build systems
- 31 12.1 Maven
- 31 Inheriting the starter parent
- 31 Using Spring Boot without the parent POM
- 32 Changing the Java version
- 32 Using the Spring Boot Maven plugin
- 32 12.2 Gradle
- 33 12.3 Ant
- 33 12.4 Starter POMs
- 36 13. Structuring your code
- 36 13.1 Using the “default” package
- 36 13.2 Locating the main application class
- 38 14. Configuration classes
- 38 14.1 Importing additional configuration classes
- 38 14.2 Importing XML configuration
- 39 15. Auto-configuration
- 39 15.1 Gradually replacing auto-configuration
- 39 15.2 Disabling specific auto-configuration
- 40 16. Spring Beans and dependency injection
- 41 17. Running your application
- 41 17.1 Running from an IDE
- 41 17.2 Running as a packaged application
- 41 17.3 Using the Maven plugin
- 42 17.4 Using the Gradle plugin
- 42 17.5 Hot swapping
- 43 18. Packaging your application for production
- 44 19. What to read next
- 45 Part IV. Spring Boot features
- 46 20. SpringApplication
- 46 20.1 Customizing the Banner
- 46 20.2 Customizing SpringApplication
- 47 20.3 Fluent builder API
- 47 20.4 Application events and listeners
- 48 20.5 Web environment
- 48 20.6 Using the CommandLineRunner
- 48 20.7 Application exit
- 49 21. Externalized Configuration
- 50 21.1 Accessing command line properties
- 50 21.2 Application property files
- 51 21.3 Profile specific properties
- 51 21.4 Placeholders in properties
- 51 21.5 Using YAML instead of Properties
- 51 Loading YAML
- 52 Exposing YAML as properties in the Spring Environment
- 52 Multi-profile YAML documents
- 53 YAML shortcomings
- 53 21.6 Typesafe Configuration Properties
- 54 Relaxed binding
- 54 @ConfigurationProperties Validation
- 55 22. Profiles
- 55 22.1 Adding active profiles
- 55 22.2 Programmatically setting profiles
- 55 22.3 Profile specific configuration files
- 56 23. Logging
- 56 23.1 Log format
- 56 23.2 Console output
- 57 23.3 File output
- 57 23.4 Custom log configuration
- 58 24. Developing web applications
- 58 24.1 The “Spring Web MVC framework”
- 58 Spring MVC auto-configuration
- 59 HttpMessageConverters
- 59 MessageCodesResolver
- 59 Static Content
- 60 Template engines
- 60 Error Handling
- 61 24.2 Embedded servlet container support
- 61 Servlets and Filters
- 61 The EmbeddedWebApplicationContext
- 61 Customizing embedded servlet containers
- 61 Programmatic customization
- 62 Customizing ConfigurableEmbeddedServletContainerFactory directly
- 62 JSP limitations
- 63 25. Security
- 65 26. Working with SQL databases
- 65 26.1 Configure a DataSource
- 65 Embedded Database Support
- 65 Connection to a production database
- 66 26.2 Using JdbcTemplate
- 66 26.3 JPA and “Spring Data”
- 67 Entity Classes
- 68 Spring Data JPA Repositories
- 68 Creating and dropping JPA databases
- 69 27. Working with NoSQL technologies
- 69 27.1 Redis
- 69 Connecting to Redis
- 69 27.2 MongoDB
- 69 Connecting to a MongoDB database
- 70 MongoTemplate
- 70 Spring Data MongoDB repositories
- 71 27.3 Gemfire
- 71 27.4 Solr
- 71 Connecting to Solr
- 71 Spring Data Solr repositories
- 72 27.5 Elasticsearch
- 72 Connecting to Elasticsearch
- 72 Spring Data Elasticsearch repositories
- 73 28. Messaging
- 73 28.1 JMS
- 73 HornetQ support
- 74 ActiveMQ support
- 74 Using JmsTemplate
- 75 29. Spring Integration
- 76 30. Monitoring and management over JMX
- 77 31. Testing
- 77 31.1 Test scope dependencies
- 77 31.2 Testing Spring applications
- 77 31.3 Testing Spring Boot applications
- 79 31.4 Test utilities
- 79 ConfigFileApplicationContextInitializer
- 79 EnvironmentTestUtils
- 79 OutputCapture
- 79 TestRestTemplate
- 81 32. Developing auto-configuration and using conditions
- 81 32.1 Understanding auto-configured beans
- 81 32.2 Locating auto-configuration candidates
- 81 32.3 Condition annotations
- 81 Class conditions
- 81 Bean conditions
- 82 Resource conditions
- 82 Web Application Conditions
- 82 SpEL expression conditions
- 83 33. What to read next
- 84 Part V. Spring Boot Actuator: Production-ready features
- 85 34. Enabling production-ready features.
- 86 35. Endpoints
- 86 35.1 Customizing endpoints
- 87 35.2 Custom health information
- 87 35.3 Custom application info information
- 88 Git commit information
- 89 36. Monitoring and management over HTTP
- 89 36.1 Exposing sensitive endpoints
- 89 36.2 Customizing the management server context path
- 89 36.3 Customizing the management server port
- 90 36.4 Customizing the management server address
- 90 36.5 Disabling HTTP endpoints
- 91 37. Monitoring and management over JMX
- 91 37.1 Customizing MBean names
- 91 37.2 Disabling JMX endpoints
- 91 37.3 Using Jolokia for JMX over HTTP
- 91 Customizing Jolokia
- 91 Disabling Jolokia
- 93 38. Monitoring and management using a remote shell
- 93 38.1 Connecting to the remote shell
- 93 Remote shell credentials
- 93 38.2 Extending the remote shell
- 94 Remote shell commands
- 94 Remote shell plugins
- 95 39. Metrics
- 95 39.1 Recording your own metrics
- 96 39.2 Metric repositories
- 96 39.3 Coda Hale Metrics
- 96 39.4 Message channel integration
- 97 40. Auditing
- 98 41. Tracing
- 98 41.1 Custom tracing
- 99 42. Process monitoring
- 99 42.1 Extend configuration
- 99 42.2 Programmatically
- 100 43. What to read next
- 101 Part VI. Deploying to the cloud
- 102 44. Cloud Foundry
- 103 44.1 Binding to services
- 104 45. Heroku
- 106 46. CloudBees
- 107 47. Openshift
- 108 48. What to read next
- 109 Part VII. Spring Boot CLI
- 110 49. Installing the CLI
- 111 50. Using the CLI
- 111 50.1 Running applications using the CLI
- 112 Deduced “grab” dependencies
- 112 Deduced “grab” coordinates
- 113 Custom “grab” metadata
- 113 Default import statements
- 113 Automatic main method
- 113 50.2 Testing your code
- 114 50.3 Applications with multiple source files
- 114 50.4 Packaging your application
- 114 50.5 Using the embedded shell
- 116 51. Developing application with the Groovy beans DSL
- 117 52. What to read next
- 118 Part VIII. Build tool plugins
- 119 53. Spring Boot Maven plugin
- 119 53.1 Including the plugin
- 120 53.2 Packaging executable jar and war files
- 121 54. Spring Boot Gradle plugin
- 121 54.1 Including the plugin
- 121 54.2 Declaring dependencies without versions
- 122 Custom version management
- 122 54.3 Default exclude rules
- 123 54.4 Packaging executable jar and war files
- 123 54.5 Running a project in-place
- 123 54.6 Spring Boot plugin configuration
- 124 54.7 Repackage configuration
- 124 54.8 Repackage with custom Gradle configuration
- 125 Configuration options
- 125 54.9 Understanding how the Gradle plugin works
- 127 55. Supporting other build systems
- 127 55.1 Repackaging archives
- 127 55.2 Nested libraries
- 127 55.3 Finding a main class
- 127 55.4 Example repackage implementation
- 128 56. What to read next
- 129 Part IX. “How-to” guides
- 130 57. Spring Boot application
- 130 57.1 Troubleshoot auto-configuration
- 130 57.2 Customize the Environment or ApplicationContext before it starts
- 131 57.3 Build an ApplicationContext hierarchy (adding a parent or root context)
- 131 57.4 Create a non-web application
- 132 58. Properties & configuration
- 132 58.1 Externalize the configuration of SpringApplication
- 132 58.2 Change the location of external properties of an application
- 132 58.3 Use “short” command line arguments
- 133 58.4 Use YAML for external properties
- 133 58.5 Set the active Spring profiles
- 134 58.6 Change configuration depending on the environment
- 134 58.7 Discover built-in options for external properties
- 135 59. Embedded servlet containers
- 135 59.1 Add a Servlet, Filter or ServletContextListener to an application
- 135 59.2 Change the HTTP port
- 135 59.3 Use a random unassigned HTTP port
- 135 59.4 Discover the HTTP port at runtime
- 136 59.5 Configure Tomcat
- 136 59.6 Terminate SSL in Tomcat
- 136 59.7 Enable Multiple Connectors Tomcat
- 137 59.8 Use Tomcat behind a front-end proxy server
- 137 59.9 Use Jetty instead of Tomcat
- 138 59.10 Configure Jetty
- 138 59.11 Use Tomcat 8
- 138 59.12 Use Jetty 9
- 140 60. Spring MVC
- 140 60.1 Write a JSON REST service
- 140 60.2 Write an XML REST service
- 140 60.3 Customize the Jackson ObjectMapper
- 141 60.4 Customize the @ResponseBody rendering
- 141 60.5 Handling Multipart File Uploads
- 141 60.6 Switch off the Spring MVC DispatcherServlet
- 141 60.7 Switch off the Default MVC configuration
- 142 60.8 Customize ViewResolvers
- 144 61. Logging
- 144 61.1 Configure Logback for logging
- 144 61.2 Configure Log4j for logging
- 146 62. Data Access
- 146 62.1 Configure a DataSource
- 146 62.2 Configure Two DataSources
- 146 62.3 Use Spring Data repositories
- 147 62.4 Separate @Entity definitions from Spring configuration
- 147 62.5 Configure JPA properties
- 147 62.6 Use a custom EntityManagerFactory
- 147 62.7 Use Two EntityManagers
- 148 62.8 Use a traditional persistence.xml
- 148 62.9 Use Spring Data JPA and Mongo repositories
- 149 63. Database initialization
- 149 63.1 Initialize a database using JPA
- 149 63.2 Initialize a database using Hibernate
- 149 63.3 Initialize a database using Spring JDBC
- 150 63.4 Initialize a Spring Batch database
- 150 63.5 Use a higher level database migration tool
- 150 Execute Flyway database migrations on startup
- 150 Execute Liquibase database migrations on startup
- 151 64. Batch applications
- 151 64.1 Execute Spring Batch jobs on startup
- 152 65. Actuator
- 152 65.1 Change the HTTP port or address of the actuator endpoints
- 152 65.2 Customize the “whitelabel” error page
- 153 66. Security
- 153 66.1 Switch off the Spring Boot security configuration
- 153 66.2 Change the AuthenticationManager and add user accounts
- 153 66.3 Enable HTTPS when running behind a proxy server
- 155 67. Hot swapping
- 155 67.1 Reload static content
- 155 67.2 Reload Thymeleaf templates without restarting the container
- 155 67.3 Reload FreeMarker templates without restarting the container
- 155 67.4 Reload Groovy templates without restarting the container
- 155 67.5 Reload Velocity templates without restarting the container
- 155 67.6 Reload Java classes without restarting the container
- 155 Configuring Spring Loaded for use with Gradle and IntelliJ
- 157 68. Build
- 157 68.1 Customize dependency versions with Maven
- 157 68.2 Create an executable JAR with Maven
- 158 68.3 Create an additional executable JAR
- 158 68.4 Extract specific libraries when an executable jar runs
- 159 68.5 Create a non-executable JAR with exclusions
- 160 68.6 Remote debug a Spring Boot application started with Maven
- 160 68.7 Remote debug a Spring Boot application started with Gradle
- 160 68.8 Build an executable archive with Ant
- 162 69. Traditional deployment
- 162 69.1 Create a deployable war file
- 162 69.2 Create a deployable war file for older servlet containers
- 162 69.3 Convert an existing application to Spring Boot
- 164 Part X. Appendices
- 165 Appendix A. Common application properties
- 171 Appendix B. Auto-configuration classes
- 171 B.1 From the “spring-boot-autoconfigure” module
- 172 B.2 From the “spring-boot-actuator” module
- 174 Appendix C. The executable jar format
- 174 C.1 Nested JARs
- 174 The executable jar file structure
- 174 The executable war file structure
- 175 C.2 Spring Boot’s “JarFile” class
- 175 Compatibility with the standard Java “JarFile”
- 175 C.3 Launching executable jars
- 176 Launcher manifest
- 176 Exploded archives
- 176 C.4 PropertiesLauncher Features
- 177 C.5 Executable jar restrictions
- 177 Zip entry compression
- 177 System ClassLoader
- 177 C.6 Alternative single jar solutions
- 178 Appendix D. Dependency versions