advertisement
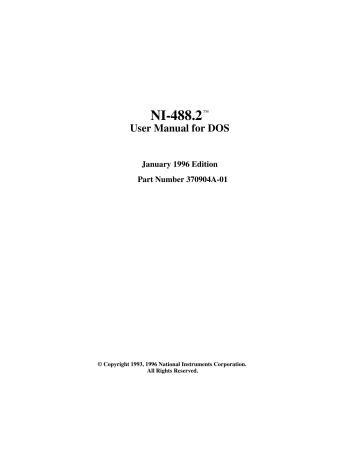
Chapter 3
Developing Your Application
This chapter explains how to develop a GPIB application program using NI-488 functions and NI-488.2 routines.
Choosing a Programming Method
Programs that need to communicate across the GPIB can access the NI-488.2 driver using either the NI-488.2 language interface or the universal language interface (ULI). If you are writing a new GPIB application program, use the NI-488.2 language interface.
Using the NI-488.2 Language Interface
Your NI-488.2 software includes two distinct sets of subroutines to meet your application needs. For most application programs, the NI-488 functions are sufficient. You should use the NI-488.2 routines if you have a complex configuration with one or more interface boards and multiple devices.
The following sections discuss some differences between NI-488 functions and NI-488.2
routines.
Using NI-488 Functions: One Device for Each Board
If your system has only one device attached to each board, the NI -488 functions are probably sufficient for your programming needs. Some other factors that make the
NI -488 functions more convenient include the following:
• With NI-488 asynchronous I/O functions ( ibcmda , ibrda , and ibwrta ), you can initiate an I/O sequence while maintaining control over the CPU for non-GPIB tasks.
• NI-488 functions include built-in file transfer functions ( ibrdf and ibwrtf ).
• With NI-488 functions, you can control the bus in non-typical ways or communicate with non-compliant devices.
The NI -488 functions consist of high-level (or device) functions that hide much of the
GPIB management operations and low-level (or board) functions that offer you more control over the GPIB than NI-488.2 routines. The following sections describe these different function types.
© National Instruments Corp.
3-1 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
NI-488 Device Functions
Device functions are high-level functions that automatically execute commands that handle bus management operations such as reading from and writing to devices or polling them for status. If you use device functions, you do not need to understand GPIB protocol or bus management. For information about device-level calls and how they manage the GPIB, refer to Device-Level Calls and Bus Management , in Chapter 7, GPIB
Programming Techniques.
NI-488 Board Functions
Board functions are low-level functions that perform rudimentary GPIB operations.
Board functions access the interface board directly and require you to handle the addressing and bus management protocol. In cases when the high-level device functions might not meet your needs, low-level board functions give you the flexibility and control to handle situations such as the following:
• Communicating with non-compliant (non-IEEE 488.2) devices
• Altering various low-level board configurations
• Managing the bus in non-typical ways
The NI-488 board functions are compatible with, and can be interspersed within, sequences of NI-488.2 routines. When you use board functions within a sequence of
NI -488.2 routines, you do not need a prior call to ibfind to obtain a board descriptor.
You simply substitute the board index as the first parameter of the board function call.
With this flexibility, you can handle non -standard or unusual situations that you cannot resolve using NI-488.2 routines only.
Using NI-488.2 Routines: Multiple Boards and/or Multiple Devices
When your system includes a board that must access more than one device, use the
NI -488.2 routines. NI-488.2 routines can perform the following tasks with a single call:
• Find all of the Listeners on the bus
• Find a device requesting service
• Determine the state of the SRQ line, or wait for SRQ to be asserted
• Address multiple devices to listen
NI-488.2 User Manual for DOS 3-2 © National Instruments Corp.
Chapter 3 Developing Your Application
Using the Universal Language Interface (ULI)
If you are writing a new GPIB application, use the NI-488.2 language interface. If you already have an existing application that uses HP-style calls, you can use the universal language interface (ULI) to access the NI-488.2 software. For more information about using the ULI, refer to Appendix C, Universal Language Interface .
Checking Status with Global Variables
Each NI-488 function and NI-488.2 routine updates the global variables to reflect the status of the device or board that you are using. The status word ( ibsta ), the error variable ( iberr ), and the count variables ( ibcnt and ibcntl ) contain useful information about the performance of your application program. Your program should check these variables frequently. The following sections describe each of these global variables and how you can use them in your application program. You can print out the values of the global variables at any time while the application is running.
Status Word – ibsta
All functions update a global status word, ibsta , which contains information about the state of the GPIB and the GPIB hardware. Most of the NI-488 functions return the value stored in ibsta . You can test for conditions reported in ibsta to make decisions about continued processing, or you can debug your program by checking ibsta after each call.
ibsta is a 16-bit value. A bit value of one (1) indicates that a certain condition is in effect. A bit value of zero (0) indicates that the condition is not in effect. Each bit in ibsta can be set for NI-488 device calls (dev), NI-488 board calls and NI-488.2 calls
(brd), or both (dev, brd).
Table 3-1 shows the condition that each bit position represents, the bit mnemonics, and the type of calls for which each bit can be set. For a detailed explanation of each of the status conditions, refer to Appendix A, Status Word Conditions .
© National Instruments Corp.
3-3 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
Mnemonic
ERR
TIMO
END
SRQI
RQS
SPOLL
Bit
Pos.
15
14
13
12
11
10
EVENT
CMPL
LOK
REM
CIC
ATN
TACS
LACS
DTAS
DCAS
3
2
5
4
1
0
7
6
9
8
20
10
8
4
200
100
80
40
2
1
Table 3-1. Status Word (ibsta) Layout
Hex
Value Type Description
8000
4000
2000
1000
800
400 dev, brd dev, brd dev, brd brd dev brd
GPIB error
Time limit exceeded
END or EOS detected
SRQ interrupt received
Device requesting service
Board has been serial polled by
Controller brd DCAS, DTAS, or IFC event has occurred dev, brd I/O completed brd brd
Lockout State
Remote State brd brd brd brd brd brd
Controller-In-Charge
Attention is asserted
Talker
Listener
Device Trigger State
Device Clear State
The language header files included on your distribution disk contain the mnemonic constants for ibsta . You can check a bit position in ibsta by using its numeric value or its mnemonic constant. For example, bit position 15 (hex 8000) detects a GPIB error.
The mnemonic for this bit is ERR. To check for a GPIB error, use either of the following statements after each NI -488 function and NI-488.2 routine as shown: if (ibsta & ERR) gpiberr(); or if (ibsta & 0x8000) gpiberr(); where gpiberr() is an error handling routine.
NI-488.2 User Manual for DOS 3-4 © National Instruments Corp.
Chapter 3 Developing Your Application
Error Variable
– iberr
If the ERR bit is set in the status word ( ibsta ), a GPIB error has occurred. When an error occurs, the error type is specified by the value in iberr .
Note:
The value in iberr is meaningful as an error type only when the ERR bit is set, indicating that an error has occurred .
For more information on error codes and solutions refer to Chapter 4, Debugging Your
Application , or Appendix B, Error Codes and Solutions.
Count Variables
– ibcnt and ibcntl
The count variables are updated after each read, write, or command function. ibcnt is a 16-bit integer and ibcntl is a 32-bit integer. If you are reading data, the count variables indicate the number of bytes read. If you are sending data or commands, the count variables reflect the number of bytes sent.
In your application program, you can use the count variables to null-terminate an ASCII string of data received from an instrument. For example, if data is received in an array of characters, you can use ibcntl to null-terminate the array and print the measurement on the screen as follows: char rdbuf[512]; ibrd (ud, rdbuf, 20L); if (!(ibsta & ERR)){ rdbuf[ibcntl] = '\0'; printf ("Read: %s\n", rdbuf);
} else { error();
} ibcntl is the number of bytes received. Data begins in the array at index zero (0); therefore, ibcntl is the position for the null character that marks the end of the string.
Using ibic to Communicate with Devices
Before you begin writing your application program, you might want to use the ibic utility. With ibic (Interface Bus Interactive Control), you communicate with your instruments from the keyboard rather than from an application program. Before you develop your GPIB application, you can use ibic to learn how to communicate with your instruments and to determine your programming needs. For specific device communication instructions, refer to the user manual that came with your instrument.
For information about using ibic and for detailed examples, refer to Chapter 5, ibic–
Interface Bus Interactive Control Utility .
© National Instruments Corp.
3-5 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
After you have learned how to communicate with your devices in ibic , you are ready to begin writing your application program.
Writing Your NI-488 Application
This section discusses items you should include in your application program, general program steps, and an NI-488 example. In this manual, the example code is presented in C using the standard C language interface. The NI -488.2 software includes the source code for this example written in C ( devsamp.c
) and the source code for this example written in BASIC ( devsamp.bas
).
The NI-488.2 software also includes the source code for nine application examples, which are described in Chapter 2, Application Examples .
Items to Include
• Include the GPIB header file. This file contains prototypes for the NI-488 functions and constants that you can use in your application program.
• Check for errors after each NI-488 function call.
• Declare and define a function to handle GPIB errors. This function takes the device offline and closes the application. If the function is declared as follows: void gpiberr (char *msg); /* function prototype */ then your application invokes the function as follows: if (ibsta & ERR) { gpiberr("GPIB error");
}
NI-488.2 User Manual for DOS 3-6 © National Instruments Corp.
Chapter 3 Developing Your Application
NI-488 Program Shell
Figure 3-1 is a flowchart of the steps to create your application program using device-level NI-488 functions.
START
Open Device (ibdev)
Are All
Devices
Open?
Yes
No
Make a Device-Level Call
• Send Data to Device (ibwrt)
• Receive Data from Device (ibrd)
• Clear Device (ibclr)
• Serial Poll Device (ibrsp)
and so on
Finished GPIB
Programming?
No
Yes
Close Device (ibonl)
Closed All
Devices?
END
Yes
No
Figure 3-1. General Program Shell Using NI-488 Device Functions
© National Instruments Corp.
3-7 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
General Program Steps and Examples
The following steps demonstrate how to use the NI-488 device functions in your program. This example configures a digital multimeter, reads 10 voltage measurements, and computes the average of these measurements.
Step 1. Open a Device
Your first NI-488 function call should be to ibdev to open a device.
ud = ibdev(0, 1, 0 , T10s, 1, 0); if (ibsta & ERR) {
gpiberr("ibdev error");
}
The input arguments of the ibdev function are as follows:
0 - board index for GPIB0
1 - primary GPIB address of the devi ce
0 - no secondary GPIB address for the device
T10s - I/O timeout value (10 s)
1 - send END message with the last byte when writing to device
0 - disable EOS detection mode
When you call ibdev , the driver automatically initializes the GPIB by sending an
Interface Clear (IFC) message and placing the device in remote programming state.
Step 2. Clear the Device
Clear the device before you configure the device for your application. Clearing the device resets its internal functions to a default state.
ibclr(ud); if (ibsta & ERR) { gpiberr("ibclr error");
}
NI-488.2 User Manual for DOS 3-8 © National Instruments Corp.
Chapter 3 Developing Your Application
Step 3. Configure the Device
After you open and clear the device, it is ready to receive commands. To configure the instrument, you send device-specific commands using the ibwrt function. Refer to the instrument user manual for the command bytes that work with your instrument.
ibwrt(ud, "*RST; VAC; AUTO; TRIGGER 2; *SRE 16", 35L); if (ibsta & ERR) { gpiberr("ibwrt error");
}
The programming instruction in this example resets the multimeter ( *RST ). The meter is instructed to measure the volts alternating current ( VAC ) using auto-ranging ( AUTO ), to wait for a trigger from the GPIB interface board before starting a measurement
( TRIGGER 2 ), and to assert the SRQ line when the measurement completes and the multimeter is ready to send the result ( *SRE 16 ).
Step 4. Trigger the Device
If you configure the device to wait for a trigger, you must send a trigger command to the device before reading the measurement value. Then instruct the device to send the next triggered reading to its GPIB output buffer.
ibtrg(ud); if (ibsta & ERR) { gpiberr("ibtrg error");
} ibwrt(ud,"VAL1?", 5L); if (ibsta & ERR) { gpiberr("ibwrt error");
}
Step 5. Wait for the Measurement
After you trigger the device, the RQS bit is set when the device is ready to send the measurement. You can detect RQS by using the ibwait function. The second parameter indicates what you are waiting for. Notice that the ibwait function also returns when the I/O timeout value is exceeded.
printf("Waiting for RQS...\n"); ibwait (ud, TIMO | RQS); if (ibsta & (ERR | TIMO)) { gpiberr("ibwait error");
}
© National Instruments Corp.
3-9 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
When SRQ has been detected, serial poll the instrument to determine if the measured data is valid or if a fault condition exists. For IEEE 488.2 instruments, you can find out by checking the message available (MAV) bit, bit 4 in the status byte that you receive from the instrument.
ibrsp (ud, &StatusByte); if (ibsta & ERR) { gpiberr("ibrsp error");
} if ( !(StatusByte & MAVbit)) { gpiberr("Improper Status Byte"); printf(" Status Byte = 0x%x\n", StatusByte);
}
Step 6. Read the Measurement
If the data is valid, read the measurement from the instrument. ( AsciiToFloat is a function that takes a null-terminated string as input and outputs the floating point number it represents.) ibrd (ud, rdbuf, 10L); if (ibsta & ERR) { gpiberr("ibrd error");
} rdbuf[ibcntl] = '\0'; printf("Read: %s\n", rdbuf);
/* Output ==> Read: +10.98E-3 */ sum += AsciiToFloat(rdbuf);
Step 7. Process the Data
Repeat steps 4 through 6 in a loop until 10 measurements have been read. Then print the average of the readings as shown: printf("The average of the 10 readings is %f\n", sum/10.0);
Step 8. Place the Device Offline
As a final step, take the device offline using the ibonl function.
ibonl (ud, 0);
NI-488.2 User Manual for DOS 3-10 © National Instruments Corp.
Chapter 3 Developing Your Application
Writing Your NI-488.2 Application
This section discusses items you should include in an application program that uses
NI -488.2 routines, general program steps, and an NI-488.2 example. In this manual the example code is presented in C using the standard C language interface. The NI-488.2
software includes the source code for this example written in C ( samp4882.c
) and the source code for this example written in BASIC ( samp488.2.bas
).
The NI-488.2 software also includes the source code for nine application examples, which are described in Chapter 2, Application Examples .
Items to Include
• Include the appropriate GPIB header file. This file contains prototypes for the
NI -488.2 routines and constants that you can use in your application program.
• Check for errors after each NI-488.2 routine.
• Declare and define a function to handle GPIB errors. This function takes the device offline and closes the application. If the function is declared as follows: void gpiberr (char *msg); /* function prototype */ then your application invokes the function as follows: if (ibsta & ERR) { gpiberr("GPIB error");
}
© National Instruments Corp.
3-11 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
NI-488.2 Program Shell
Figure 3-2 is a flowchart of the steps to create your application program using NI-488.2
routines.
START
Initialize Specified GPIB
Interface ( SendIFC )
Low-Level
Are All Boards
Initialized?
Yes
No
Making
High-Level or
Low-Level Call?
High-Level
Make a Low-Level Call
• Address Devices to Listen ( SendSetup )
• Send Data to Addressed Listener
( SendDataBytes )
• Address Device to Talk ( ReceiveSetup )
• Receive Data from Addressed Talker
( RcvRespMsg )
and so on
Make a High-Level Call
• Send Data to Device ( Send )
• Receive Data from Device
( Receive )
• Clear Device ( DevClear )
• Serial Poll Device
( ReadStatusByte )
and so on
Finished GPIB
Programming?
Yes
No
Close Board
( ibonl )
Are All Boards
Closed?
No
END
Yes
Figure 3-2. General Program Shell Using NI-488.2 Routines
NI-488.2 User Manual for DOS 3-12 © National Instruments Corp.
Chapter 3 Developing Your Application
General Program Steps and Examples
The following steps demonstrate how to use the NI-488.2 routines in your program. This example configures a digital multimeter, reads 10 voltage measurements, and computes the average of these measurements.
Step 1. Initialization
Use the SendIFC routine to initialize the bus and the GPIB interface board so that the
GPIB board is Controller-In-Charge (CIC). The only argument of SendIFC is the GPIB interface board number.
SendIFC(0); if (ibsta & ERR) { gpiberr("SendIFC error");
}
Step 2. Find All Listeners
Use the FindLstn routine to create an array of all of the instruments attached to the
GPIB. The first argument is the interface board number, the second argument is the list of instruments that was created, the third argument is a list of instrument addresses that the procedure actually found, and the last argument is the maximum number of devices that the procedure can find (that is, it must stop if it reaches the limit). The end of the list of addresses must be marked with the NOADDR constant, which is defined in the header file that you included at the beginning of the program.
for (loop = 0; loop <=30; loop++){ instruments[loop] = loop;
} instruments[31] = NOADDR; printf("Finding all Listeners on the bus...\n");
Findlstn(0, instruments, result, 30); if (ibsta & ERR) { gpiberr ("FindLstn error");
}
Step 3. Identify the Instrument
Send an identification query to each device for identification. For this example, assume that all of the instruments are IEEE 488.2-compatible and can accept the identification query, *IDN?
. In addition, assume that FindLstn found the GPIB interface board at primary address 0 (default) and, therefore, you can skip the first entry in the result array.
© National Instruments Corp.
3-13 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3 for (loop = 1; loop <= num_Listeners; loop++) {
Send(0, result[loop], "*IDN?", 5L, NLend); if (ibsta & ERR) { gpiberr("Send error");
}
Receive(0, result[loop], buffer, 10L, STOPend); if (ibsta & ERR) { gpiberr("Receive error");
} buffer[ibcntl] = '\0'; printf("The instrument at address %d is a %s\n",
result[loop], buffer); if (strncmp(buffer, "Fluke, 45", 9) == 0) { fluke = result[loop]; printf("**** Found the Fluke ****\n"); break;
}
} if (loop > num_Listeners) { printf("Did not find the Fluke!\n"); ibonl(0,0); exit(1);
}
The constant NLend signals that the new line character with EOI is automatically appended to the data to be sent.
The constant STOPend indicates that the read is stopped when EOI is detected.
Step 4. Initialize the Instrument
After you find the multimeter, use the DevClear routine to clear it. The first argument is the GPIB board number. The second argument is the GPIB address of the multimeter.
Then send the IEEE 488.2 reset command to the meter.
DevClear(0, fluke); if (ibsta & ERR) { gpiberr("DevClear error")
}
Send(0, fluke, "*RST", 4L, NLend); if (ibsta & ERR) { gpiberr("Send *RST error");
} sum = 0.0; for(m =0; m<10; m++){
/* start of loop for Steps 5 through 8 */
NI-488.2 User Manual for DOS 3-14 © National Instruments Corp.
Chapter 3 Developing Your Application
Step 5. Configure the Instrument
After initialization, the instrument is ready to receive instructions. To configure the multimeter, use the Send routine to send device-specific commands. The first argument is the number of the access board. The second argument is the GPIB address of the multimeter. The third argument is a string of bytes to send to the multimeter.
The bytes in this example instruct the meter to measure volts alternating current ( VAC ) using auto-ranging ( AUTO ), to wait for a trigger from the Controller before starting a measurement ( TRIGGER 2 ), and to assert SRQ when the measurement has been completed and the meter is ready to send the result ( *SRE 16 ). The fourth argument represents the number of bytes to be sent. The last argument, NLend , is a constant defined in the header file which tells Send to append a linefeed character, with EOI asserted, to the end of the message sent to the multimeter.
Send (0, fluke, "VAC; AUTO; TRIGGER 2; *SRE 16", 29L, NLend); if (ibsta & ERR) { gpiberr("Send setup error");
}
Step 6. Trigger the Instrument
In the previous step, the multimeter was instructed to wait for a trigger before conducting a measurement. Now send a trigger command to the multimeter. You could use the
Trigger routine to accomplish this, but because the Fluke 45 is IEEE 488.2compatible, you can just send it the trigger command, *TRG.
The VAL1?
command instructs the meter to send the next triggered reading to its output buffer.
Send(0, fluke, "*TRG; VAL1?", 11L, NLend); if (ibsta & ERR) { gpiberr("Send trigger error");
}
Step 7. Wait for the Measurement
After the meter is triggered, it takes a measurement and displays it on its front panel and then asserts SRQ. You can detect the assertion of SRQ using either the TestSRQ or
WaitSRQ routine. If you have a process that you want to execute while you are waiting for the measurement, use TestSRQ . For this example, you can use the WaitSRQ routine. The first argument in WaitSRQ is the GPIB board number. The second argument is a flag returned by WaitSRQ that indicates whether or not SRQ is asserted.
WaitSRQ(0, &SRQasserted); if (!SRQasserted) { gpiberr("WaitSRQ error");
}
© National Instruments Corp.
3-15 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
After you have detected SRQ, use the ReadStatusByte routine to poll the meter and determine its status. The first argument is the GPIB board number, the second argument is the GPIB address of the instrument, and the last argument is a variable that
ReadStatusByte uses to store the status byte of the instrument.
ReadStatusByte(0, fluke, &statusByte); if (ibsta & ERR) { gpiberr("ReadStatusByte error");
}
After you have obtained the status byte, you must check to see if the meter has a message to send. You can do this by checking the message available (MAV) bit, bit 4 in the status byte.
if (!(statusByte & MAVbit) { gpiberr("Improper Status Byte"); printf("Status Byte = 0x%x\n", statusByte);
}
Step 8. Read the Measurement
Use the Receive function to read the measurement over the GPIB. The first argument is the GPIB interface board number, and the second argument is the GPIB address of the multimeter. The third argument is a string into which the Receive function places the data bytes from the multimeter. The fourth argument represents the number of bytes to be received. The last argument indicates that the Receive message terminates upon receiving a byte accompanied with the END message.
Receive(0, fluke, buffer, 10L, STOPend); if (ibsta & ERR) { gpiberr("Receive error");
} buffer[ibcntl] = '\0'; printf (Reading : %s\n", buffer); sum += AsciiToFloat(buffer);
} /* end of loop started in Step 5 */
Step 9. Process the Data
Repeat Steps 5 through 8 in a loop until 10 measurements have been read. Then print the average of the readings as shown: printf (" The average of the 10 readings is : %f\n", sum/10);
NI-488.2 User Manual for DOS 3-16 © National Instruments Corp.
Chapter 3 Developing Your Application
Step 10. Place the Board Offline
Before ending your application program, take the board offline using the ibonl function.
ibonl(0,0);
Compiling, Linking, and Running Your C Application
This section describes how to compile, link, and run your application program. To see examples that show how to include specific lines of code in your program, refer to the program examples included with your NI-488.2 software.
Before you compile your application program, make sure that the following line is included at the beginning of your program:
#include "decl.h"
After you have written your Microsoft C application program, you need to compile your application program using the Microsoft C (version 5.1 or higher) compiler. Then link your application with the C language interface, mcib.lib
. Use the following command to compile and link a C application named cprog using Microsoft C: cl cprog.c mcib.lib
After you have written your Borland C++ application, you need to compile your application program using the Borland C++ (version 2.0 or higher) compiler. Then link your application with the C language interface, mcib.lib
. Use the following command to compile and link an application named cprog using Borland C++: bcc cprog.c mcib.lib
To run your Microsoft C or Borland C++ application, enter the following command: cprog
If you discover errors when you execute the program, refer to Chapter 4, Debugging Your
Application . If you want to verify the NI-488 and NI-488.2 calls made by your application, you can use appmon , the GPIB Applications Monitor described in
Chapter 6, appmon–GPIB Applications Monitor .
© National Instruments Corp.
3-17 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
Compiling, Linking, and Running Your BASIC Application
The NI-488.2 software includes language interfaces for Microsoft Professional BASIC
(version 7.0 or higher), Microsoft Visual Basic (version 1.0), QuickBASIC (version 4.0
or higher), BASICA, and GWBASIC.
The following sections describe how to compile, link, and run your application program.
To see examples that show how to include specific lines of code in your program, refer to the program examples included with your NI-488.2 software.
With the BASIC languages, you access the NI-488 functions as subroutines, using the
BASIC keyword CALL followed by the NI-488 function name (for example CALL ibtrg (ud%) ). With QuickBASIC, BASIC, or Visual Basic for DOS, you can access the NI-488 functions using another set of functions, the il functions (for example result% = iltrg (ud%) ). If you prefer calling functions instead of subroutines, then you can use the il functions. For the BASIC languages, with some of the ib commands (for example, ibrd ) the length of the string buffer is automatically calculated within the actual subroutine, which eliminates the need to pass in the length as an extra parameter.
If you discover errors when you execute the program, refer to Chapter 4, Debugging Your
Application . If you want to verify the NI-488 and NI-488.2 calls made by your application, you can use appmon , the GPIB Applications Monitor described in
Chapter 6, appmon–GPIB Applications Monitor .
Microsoft BASIC
Before you compile your application program, make sure that the following line is included at the beginning of your program:
‘$include: ‘mbdecl.bas’
After you have written your BASIC application program, you need to compile it using the Microsoft Professional BASIC (version 7.0 or higher) compiler. Then link your application with the BASIC language interface, mbib.obj
.
Using the QBX Environment
Use the following commands to compile, link, and run a BASIC program named bprog from within the QBX environment.
To create an object module library called mbib.lib
, enter the following command on the DOS command line: lib mbib.lib + mbib.obj;
NI-488.2 User Manual for DOS 3-18 © National Instruments Corp.
Chapter 3 Developing Your Application
To create a QuickLibrary called mbib.qlb
that is linked with the mbib.obj
language interface, enter the following command on the DOS command line: link /q mbib.obj, mbib.qlb,, qbxqlb.lib;
To run the program from within the QBX environment, type the following on the DOS command line: qbx bprog /l mbib.qlb
Then select
Start
from the
Run
menu.
Using the DOS Command Line
Use the following commands to compile, link, and run a BASIC program named bprog from the DOS command line.
To compile the BASIC program, enter the following command on the DOS command line: bc bprog;
To link the BASIC program with the mbib.obj
language interface, enter the following command on the DOS command line: link bprog mbib;
To run the program from the DOS command line, type: bprog
Microsoft Visual Basic
Before you compile and link your program in Visual Basic, you must include the following line in the module-level code:
'$include: 'mbdecl.bas'
Do not list mbdecl.bas
in a project .mak
file.
Use the following command to prepare the BASIC language interface, mbib.obj
, for the Visual Basic environment: lib vbib.lib + mbib.obj + vbdos.lib; link /q mbib.obj vbdos.lib,vbib.qlb,,vbdosqlb.lib;
© National Instruments Corp.
3-19 NI-488.2 User Manual for DOS
Developing Your Application Chapter 3
Enter the following command to run a Visual Basic program named bprog in the Visual
Basic environment: vbdos bprog /l vbib.qlb
Then select the
Start
option from the
Run
menu.
QuickBASIC
Before you compile your application program, make sure that the following line is included at the beginning of your program:
‘$include: ‘qbdecl.bas’
After you have written your QuickBASIC application program, you need to compile it using the Microsoft QuickBASIC (version 4.0 or higher) compiler. Then link your application with the QuickBASIC language interface, qbib.obj
.
Using the QuickBASIC Interactive Environment
Use the following commands to compile, link, and run a QuickBASIC program named bprog from the QuickBASIC interactive environment.
To create an object module library called qbib.lib
, enter the following command on the DOS command line: lib qbib.lib + qbib.obj;
To create a QuickLibrary called qbib.qlb
that is linked with the qbib.obj
language interface, enter one of the following commands on the DOS command line.
For QuickBASIC 4.0 compilers, type: link /q qbib.obj, qbib.qlb,, bqlb40.lib; or
For QuickBASIC 4.5 compilers, type: link /q qbib.obj, qbib.qlb,, bqlb45.lib;
To run the program from within the QuickBASIC interactive environment, type the following on the DOS command line: qb bprog /l qbib.qlb
Then select
Start
from the
Run
menu.
NI-488.2 User Manual for DOS 3-20 © National Instruments Corp.
Chapter 3 Developing Your Application
Using the DOS Command Line
Use the following commands to compile, link, and run a QuickBASIC program named bprog from the DOS command line.
To compile the BASIC program, enter the following command on the DOS command line: bc bprog;
To link the BASIC application program with the qbib.obj
language interface, enter the following command on the DOS command line: link bprog qbib;
To run the program from the DOS command line, type: bprog
BASICA/GWBASIC
The BASICA language interface, bib.m
, must be in the directory currently in use.
Use the following code to run a BASICA program named bprog : load "bprog.bas
merge "decl.bas
run
The clear statement in line 1 of decl.bas
contains a constant that represents the amount of memory that can be used after loading the BASICA language interface, bib.m
For most users, this constant is correct. But, if you invoke BASICA and it reports that there are fewer than 59 KB free, you need to adjust the constant to a smaller value.
© National Instruments Corp.
3-21 NI-488.2 User Manual for DOS
advertisement
Related manuals
advertisement
Table of contents
- 1 NI-488.2 User Manual for DOS
- 3 Limited Warranty
- 4 Copyright
- 4 Trademarks
- 4 WARNING REGARDING MEDICAL AND CLINICAL USE OF NATIONAL INSTRUMENTS PRODUCTS
- 5 Contents
- 12 About This Manual
- 12 How to Use This Manual Set
- 13 Organization of This Manual
- 14 Conventions Used in This Manual
- 15 Related Documentation
- 15 Customer Communication
- 16 Chapter 1 Introduction
- 16 GPIB Overview
- 16 Talkers,Listeners,and Controllers
- 16 Controller-In-Charge and System Controller
- 17 GPIB Addressing
- 17 Sending Messages Across the GPIB
- 17 Data Lines
- 18 Handshake Lines
- 18 Interface Management Lines
- 19 Setting Up and Configuring Your System
- 20 Controlling More Than One Board
- 20 Configuration Requirements
- 21 The NI-488.2 Software Components
- 21 NI-488.2 Driver and Driver Utilities
- 22 C Language Files
- 22 BASIC Language Files
- 23 Universal Language Interface File
- 23 Sample Application Files
- 23 How the NI-488.2 Software Works with DOS
- 24 Unloading and Reloading the NI-488.2 Driver
- 26 Chapter 2 Application Examples
- 27 Example 1:Basic Communication
- 29 Example 2:Clearing and Triggering Devices
- 31 Example 3:Asynchronous I/O
- 33 Example 4:End-of-String Mode
- 35 Example 5:Service Requests
- 39 Example 6:Basic Communication with IEEE 488.2-Compliant Devices
- 41 Example 7:Serial Polls Using NI-488.2 Routines
- 43 Example 8:Parallel Polls
- 45 Example 9:Non-Controller Example
- 47 Chapter 3 Developing Your Application
- 47 Choosing a Programming Method
- 47 Using the NI-488.2 Language Interface
- 47 Using NI-488 Functions:One Device for Each Board
- 48 Using NI-488.2 Routines:Multiple Boards and/or Multiple Devices
- 49 Using the Universal Language Interface (ULI)
- 49 Checking Status with Global Variables
- 49 Status Word – ibsta
- 51 Error Variable –iberr
- 51 Count Variables –ibcnt and ibcntl
- 51 Using ibic to Communicate with Devices
- 52 Writing Your NI-488 Application
- 52 Items to Include
- 53 NI-488 Program Shell
- 54 General Program Steps and Examples
- 54 Step 1.Open a Device
- 54 Step 2.Clear the Device
- 55 Step 3.Configure the Device
- 55 Step 4.Trigger the Device
- 55 Step 5.Wait for the Measurement
- 56 Step 6.Read the Measurement
- 56 Step 7.Process the Data
- 56 Step 8.Place the Device Offline
- 57 Writing Your NI-488.2 Application
- 57 Items to Include
- 58 NI-488.2 Program Shell
- 59 General Program Steps and Examples
- 59 Step 1.Initialization
- 59 Step 2.Find All Listeners
- 59 Step 3.Identify the Instrument
- 60 Step 4.Initialize the Instrument
- 61 Step 5.Configure the Instrument
- 61 Step 6.Trigger the Instrument
- 61 Step 7.Wait for the Measurement
- 62 Step 8.Read the Measurement
- 62 Step 9.Process the Data
- 63 Step 10.Place the Board Offline
- 63 Compiling,Linking,and Running Your C Application
- 64 Compiling,Linking,and Running Your BASIC Application
- 64 Microsoft BASIC
- 64 Using the QBX Environment
- 65 Using the DOS Command Line
- 65 Microsoft Visual Basic
- 66 QuickBASIC
- 66 Using the QuickBASIC Interactive Environment
- 67 Using the DOS Command Line
- 67 BASICA/GWBASIC
- 68 Chapter 4 Debugging Your Application
- 68 Running ibtest
- 68 Presence Test of Driver
- 68 Presence Test of Board
- 69 GPIB Cables Connected
- 69 ULI Driver Loaded
- 69 Running GPIBInfo
- 71 Debugging with the Global Status Variables
- 71 Debugging with ibic
- 71 Debugging with appmon
- 71 GPIB Error Codes
- 72 Configuration Errors
- 73 Timing Errors
- 73 Communication Errors
- 73 Repeat Addressing
- 74 Termination Method
- 74 Common Questions
- 76 Chapter 5 ibic –Interface Bus Interactive Control Utility
- 76 Overview
- 76 Example Using NI-488 Functions
- 79 ibic Syntax
- 79 Number Syntax
- 79 String Syntax
- 79 Address Syntax
- 79 ibic Syntax for NI-488 Functions
- 82 ibic Syntax for NI-488.2 Routines
- 83 Status Word
- 84 Error Information
- 84 Count
- 84 Common NI-488 Functions
- 84 ibfind
- 85 ibdev
- 86 ibwrt
- 86 ibrd
- 87 Common NI-488.2 Routines in ibic
- 87 Set
- 87 Send and SendList
- 87 Receive
- 88 Auxiliary Functions
- 88 Set (Select Device or Board)
- 89 Help (Display Help Information)
- 89 !(Repeat Previous Function)
- 89 -(Turn Display Off)and +(Turn Display On)
- 90 n*(Repeat Function n Times)
- 90 $(Execute Indirect File)
- 90 Print (Display the ASCII String)
- 91 Buffer (Set Buffer Display Mode)
- 92 Chapter 6 appmon –GPIB Applications Monitor
- 92 Overview
- 92 Installing appmon
- 92 Configuring appmon
- 95 Using appmon
- 96 Using the Command Keys
- 96 Viewing the GPIB History Screen
- 96 Hiding and Showing the Applications Monitor
- 97 Chapter 7 GPIB Programming Techniques
- 97 Termination of Data Transfers
- 98 High-Speed Data Transfers (HS488)
- 98 Enabling HS488
- 99 System Configuration Effects on HS488
- 99 Waiting for GPIB Conditions
- 99 Device-Level Calls and Bus Management
- 100 Talker/Listener Applications
- 100 Waiting for Messages from the Controller
- 100 Using the Event Queue
- 101 Requesting Service
- 101 Simulating Multiple Addresses
- 101 Serial Polling
- 101 Service Requests from IEEE 488 Devices
- 102 Service Requests from IEEE 488.2 Devices
- 102 Automatic Serial Polling
- 102 Stuck SRQ State
- 103 Autopolling and Interrupts
- 103 “ON SRQ ” Functionality
- 103 C “ON SRQ ” Capability
- 103 BASIC/QuickBASIC/BASICA “ON SRQ ” Capability
- 104 SRQ and Serial Polling with NI-488 Device Functions
- 104 SRQ and Serial Polling with NI-488.2 Routines
- 105 Example 1:Using FindRQS
- 106 Example 2:Using AllSpoll
- 106 Parallel Polling
- 106 Implementing a Parallel Poll
- 107 Parallel Polling with NI-488 Functions
- 108 Parallel Polling with NI-488.2 Routines
- 110 Chapter 8 ibconf –Interface Bus Configuration Utility
- 110 Overview
- 110 Starting ibconf
- 112 Upper and Lower Levels of ibconf
- 112 Upper-Level Device Map
- 115 Lower-Level Device/Board Characteristics
- 116 Board and Device Configuration Options
- 116 Primary GPIB Address
- 117 Secondary GPIB Address
- 117 Timeout Setting
- 117 Serial Poll Timeouts (Device Characteristic Only)
- 117 Terminate Read on EOS
- 118 Set EOI with EOS on Writes
- 118 Type of Compare on EOS
- 118 EOS Byte
- 118 Send EOI at End of Write
- 118 System Controller (Board Characteristic Only)
- 119 Assert REN when SC (Board Characteristic Only)
- 119 Enable Auto Serial Polling (Board Characteristic Only)
- 119 Enable CIC Protocol (Board Characteristic Only)
- 119 Bus Timing (Board Characteristic Only)
- 119 Cable Length for High Speed (Board Characteristic Only)
- 120 Parallel Poll Duration (Board Characteristic Only)
- 120 Use This GPIB Interface (Board Characteristic Only)
- 120 Base I/O Address (Board Description Only)
- 120 DMA Channel (Board Characteristic Only)
- 120 Interrupt Jumper Setting (Board Characteristic Only)
- 121 Enable Repeat Addressing (Device Characteristic Only)
- 121 GPIB-PCII/IIA Mode Switch
- 121 Default Configurations in ibconf
- 122 Exiting ibconf
- 122 Checking for Errors
- 123 Appendix A Status Word Conditions
- 128 Appendix B Error Codes and Solutions
- 136 Appendix C Universal Language Interface
- 161 Appendix D Customer Communication
- 166 Glossary
- 174 Index
- 10 Figures
- 17 Figure 1-1.GPIB Address Bits
- 19 Figure 1-2.Linear and Star System Configuration
- 20 Figure 1-3.Example of Multiboard System Setup
- 24 Figure 1-4.How the NI-488.2 Software Works with DOS
- 28 Figure 2-1.Program Flowchart for Example 1
- 30 Figure 2-2.Program Flowchart for Example 2
- 32 Figure 2-3.Program Flowchart for Example 3
- 34 Figure 2-4.Program Flowchart for Example 4
- 37 Figure 2-5.Program Flowchart for Example 5
- 38 Figure 2-5.Program Flowchart for Example 5 (Continued)
- 40 Figure 2-6.Program Flowchart for Example 6
- 42 Figure 2-7.Program Flowchart for Example 7
- 44 Figure 2-8.Program Flowchart for Example 8
- 46 Figure 2-9.Program Flowchart for Example 9
- 53 Figure 3-1.General Program Shell Using NI-488 Device Functions
- 58 Figure 3-2.General Program Shell Using NI-488.2 Routines
- 95 Figure 6-1.appmon Pop-up Screen
- 112 Figure 8-1.Upper Level of ibconf
- 115 Figure 8-2.Lower Level of ibconf
- 140 Figure C-1.Character Count Not Included in the OUTPUT Statement
- 142 Figure C-2.Character Count Included in the ENTER Statement
- 144 Figure C-3.Character Count Not Included in the ENTER Statement
- 11 Tables
- 18 Table 1-1.GPIB Handshake Lines
- 18 Table 1-2.GPIB Interface Management Lines
- 50 Table 3-1. Status Word (ibsta) Layout
- 72 Table 4-1.GPIB Error Codes
- 80 Table 5-1.Syntax for Device-Level NI-488 Functions in ibic
- 81 Table 5-2.Syntax for Board-Level NI-488 Functions in ibic
- 82 Table 5-3.Syntax for NI-488.2 Routines in ibic
- 88 Table 5-4.Auxiliary Functions in ibic
- 93 Table 6-1.ibtrap Mask Options
- 94 Table 6-2.ibtrap Monitor Mode Options
- 96 Table 6-3.Function Keys in appmon
- 111 Table 8-1.Options for Starting ibconf
- 123 Table A-1.Status Word Bits
- 128 Table B-1.GPIB Error Codes
- 145 Table C-1.Universal Language Interface Functions
- 159 Table C-2.ULI Timeout Code Values