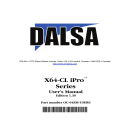
advertisement
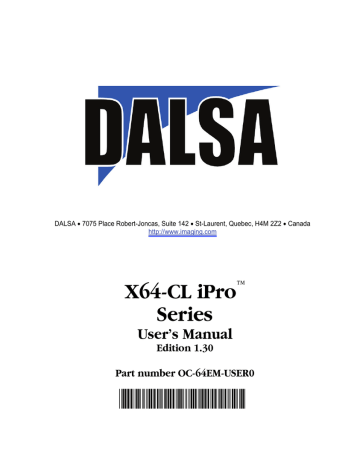
X-I/O Module Sapera Interface
Sapera version 5.30 (or later) provides support for the X-I/O module via an I/O class and demonstration program. Users can use the demonstration program as is, or use the demo program source code to implement X-I/O controls within the custom imaging application.
This section describes configuring the X-I/O module power up state, using the X-I/O demo program, and describes the Sapera Class to program and read the X-I/O module along with sample code.
Configuring User Defined Power-up I/O States
The X-I/O module power up state is stored onboard in flash memory. User configuration of this initial state is performed by the Device Manager program. Run the program via the windows start menu: (Start
• Programs • DALSA • X64-CL iPro Device Driver • Device Manager).
The Device Manager provides information on the installed X64-CL iPro board and its firmware. With an
X-I/O module installed, click on XIO Board – Information, as shown in the following figure.
116 • Appendix: X-I/O Module Option
X64-CL iPro Series User's Manual
The XIO information screen shows the current status of Device 0—the output device, and Device 1—the input device. A few items are user configurable for X-I/O board power up state. Click on the item to display a drop list of available capabilities, as described below.
• Device 0 – Default Output Type choose Tristate mode (i.e. output disconnected), or PNP mode, or NPN mode.
• Device 0 – Default Output Pin State
A window is displayed to select a logic low or high state for each output pin. Click on each pin that should be logic high by default.
• Device 1 – Default Input Level
Select the input logic level as TTL 5 Volts or 24 Volts, dependent on the signal type being input to the X-I/O module.
• Device 1 – Default Connector
DB37 is the supported output connector, as described in this section.
Programming the User Configuration
After changing any user configurable X-I/O mode from the factory default state, click on the Program button (located on the upper left), to write the new default state to flash memory. The Device Manager message output window will display "Successfully updated EEProm". The program can now be closed.
Using Sapera LT General I/O Demo
The Sapera General I/O demo program controls the I/O capabilities of the X-I/O module on the Sapera board product. The demo will present to the user only the controls pertaining to the selected hardware (in the case of multiple installed boards).
Run the demo via the windows start menu: (Start • Programs • DALSA • Sapera LT • Demos •
General I/O Demo). The first menu presents a drop list of all installed Sapera Acquisition Devices with
I/O capabilities. In the following figure the X64-CL iPro board is selected. Click OK to continue.
X64-CL iPro Series User's Manual
Appendix: X-I/O Module Option • 117
General I/O Module Control Panel
The I/O module control demo presents the I/O capabilities of the installed hardware. The following figure shows the X-I/O module connected to the X64-CL iPro board.
Output Pins: The first column displays the current state of the eight output pins (I/O Device #0).
• The startup default state is user configured using the Device Manager program.
• The state of each output can be changed by clicking on its status button.
• Use the Signal Output drop menu to select the output mode (Tristate, PNP, NPN).
Input Pins: The second section provides input pin status (I/O device #1). Note that this program is a
demo, therefore no action takes place on an input event.
• The first column reads the logic level present on each input. The Input Level drop menu changes the logic level from 5V TTL to 24V logic. Use the Device Manager program to select the default logic level type.
• The second column demonstrates activating interrupts on individual inputs. In this demo program, use the Enable box to activate the interrupt on an input. The Count box will tally detected input events. Use the Signal Event drop menu to select which input signal edge to detect. The Reset button clears all event counts.
118 • Appendix: X-I/O Module Option
X64-CL iPro Series User's Manual
X64-CL iPro Series User's Manual
Appendix: X-I/O Module Option • 119
Sapera LT General I/O Demo Code Samples
The following source code was extracted from the General I/O demo program. The comments highlight the areas that an application developer needs for embedding X-I/O module controls within the imaging application.
Main I/O Demo code
BOOL CGioMainDlg::OnInitDialog()
{
[ . . . ]
// some declarations
UINT32 m_gioCount; int m_ServerIndex; int m_ResourceIndex;
// Show the Server Dialog to select the acquisition device
CGioServer dlg(this); if (dlg.DoModal() == IDOK)
{ m_ServerIndex = dlg.GetServerIndex(); m_ServerName = dlg.GetServerName(); if ( m_ServerIndex != -1)
{
// Get the number of resources from SapManager for ResourceGio type by using
// - the server index chosen in the dialog box
// - the resource type to enquire for Gio
m_gioCount=SapManager::GetResourceCount(m_ServerIndex,SapManager::ResourceGio);
// Create all objects [see the function following] if (!CreateObjects()) { EndDialog(TRUE); return FALSE; }
[ . . . ]
//Loop for all resources for (UINT32 iDevice = 0; (iDevice < MAX_GIO_DEVICE) && (iDevice < m_gioCount);
iDevice++)
{
[ . . . ]
// direct read access to low-level Sapera C library capability to check
// I/O Output module
(m_pGio[iDevice]->IsCapabilityValid(CORGIO_CAP_DIR_OUTPUT))
status
// direct read access to low-level Sapera C library capability to
// check I/O Input module
(m_pGio[iDevice]->IsCapabilityValid(CORGIO_CAP_DIR_INPUT))
120 • Appendix: X-I/O Module Option
X64-CL iPro Series User's Manual
status
[ . . . ]
// Constructor used for I/O Output module dialog.
if
{ m_pDlgOutput[iDevice] = new CGioOutputDlg(this, iDevice, m_pGio[iDevice]);
}
[ . . . ]
// Constructor used for I/O Input module dialog. if (capInput)
{ m_pDlgInput[iDevice] = new CGioInputDlg(this, iDevice, m_pGio[iDevice]);
}
} //end for
} // end if
[ . . . ]
}
Function CreateObjects()
BOOL CreateObjects()
{
CWaitCursor wait;
// Loop for all I/O resources for (UINT32 iDevice = 0; (iDevice < MAX_GIO_DEVICE) && (iDevice < m_gioCount);
iDevice++)
{
// The SapLocation object specifying the server where the I/O resource is located
SapLocation location(m_ServerIndex, iDevice);
// The SapGio constructor is called for each resource found. m_pGio[iDevice] = new SapGio(location);
// Creates all the low-level Sapera resources needed by the I/O object if (m_pGio[iDevice] && !*m_pGio[iDevice] && !m_pGio[iDevice]->Create())
{
DestroyObjects();
FALSE;
}
} return TRUE;
}
X64-CL iPro Series User's Manual
Appendix: X-I/O Module Option • 121
Output Dialog: CGioOutputDlg class (see Sapera Gui class)
void CGioOutputDlg::UpdateIO()
{
UINT32
UINT32
BOOL status;
[ . . . ]
// We loop to get all I/O pins. for (UINT32 iIO=0; iIO < (UINT32)m_pGio->GetNumPins(); iIO++)
{
[ . . . ]
// We set the current state of the current I/O pin by using
// - the pin number on the current I/O resource
// - the pointer to pin state
// ( SapGio ::PinLow if low and SapGio ::PinHigh if high) status = m_pGio->SetPinState(iIO, (SapGio::PinState)state);
}
}
Input Dialog: CGioInputDlg class. (see Sapera Gui class)
BOOL CGioInputDlg::Update()
{
SapGio::PinState state = SapGio::PinState::PinLow;
BOOL status = true;
UINT32 jIO; iIO;
UINT32 if (m_pGio == NULL)
return
// We loop to get all I/O pins. for (iIO=0; iIO < (UINT32)m_pGio->GetNumPins(); iIO++)
{
m_pGio->SetDisplayStatusMode(SapManager::StatusLog,
// We get the current state of the current I/O pin by using
// the pin number on the current I/O resource
// the pointer to pin state
// ( SapGio ::PinLow if low and SapGio ::PinHigh if high) status = m_pGio->GetPinState(iIO, &state);
NULL);
[ . . . ]
}
[ . . . ]
}
122 • Appendix: X-I/O Module Option
X64-CL iPro Series User's Manual
I/O Event Handling
void CGioInputDlg::GioCallbackInfo(SapGioCallbackInfo *pInfo)
{
CGioInputDlg* pInputDlg;
CString strEventCount;
// We get the application context associated with I/O events pInputDlg = (CGioInputDlg*)pInfo->GetContext();
// We get the current count of I/O events
strEventCount.Format("%d",
// We get the I/O pin number that generated an I/O event and apply the changes.
pInputDlg->m_GioEventCount[pInfo->GetPinNumber()]++;
}
X64-CL iPro Series User's Manual
Appendix: X-I/O Module Option • 123
124 • Appendix: X-I/O Module Option
X64-CL iPro Series User's Manual
advertisement
Related manuals
advertisement
Table of contents
- 7 Overview of the Manual
- 8 About the Manual
- 8 Using the Manual
- 9 Product Part Numbers
- 11 About the X64-CL iPro Series of Frame Grabbers
- 11 X64-CL iPro Series Key Features
- 11 X64-CL iPro User Programmable Configurations
- 12 ACUPlus: Acquisition Control Unit
- 12 DTE: Intelligent Data Transfer Engine
- 13 Advanced Controls Overview
- 13 About the X-I/O Module
- 14 Development Software Overview
- 14 Sapera LT Library
- 14 Sapera Processing Library
- 15 Warning! (Grounding Instructions)
- 15 Upgrading Sapera or any DALSA Board Driver
- 15 Board Driver Upgrade Only
- 16 Sapera and Board Driver Upgrades
- 16 Sapera LT Library Installation
- 17 Installing X64-CL iPro Hardware and Driver
- 17 In a Windows XP, Windows Vista or Windows 7 System
- 18 X64-CL iPro Firmware Loader
- 18 Automatic Mode
- 19 Manual Mode
- 19 Executing the Firmware Loader from the Start Menu
- 20 Enabling the Camera Link Serial Control Port
- 20 COM Port Assignment
- 21 Setup Example with Windows HyperTerminal
- 22 Displaying X64-CL iPro Board Information
- 22 Device Manager – Board Information
- 23 Camera to Camera Link Connections
- 25 Configuring Sapera
- 25 Viewing Installed Sapera Servers
- 25 Increasing Contiguous Memory for Sapera Resources
- 26 Contiguous Memory for Sapera Messaging
- 27 Troubleshooting Installation Problems
- 27 Recovering from a Firmware Update Error
- 28 Windows Event Viewer
- 28 Device Manager Program
- 29 Information Window
- 30 PCI Configuration
- 31 Sapera and Hardware Windows Drivers
- 32 Log Viewer
- 33 Windows Device Manager
- 33 Memory Requirements with Area Scan Acquisitions
- 34 Symptoms: CamExpert Detects no Boards
- 34 Troubleshooting Procedure
- 35 Symptoms: X64-CL iPro Does Not Grab
- 35 Symptoms: Card grabs black
- 35 Symptoms: Card acquisition bandwidth is less than expected
- 37 Interfacing Cameras with CamExpert
- 37 CamExpert Demonstration and Test Tools
- 38 Camera Types & Files Applicable to the X64-CL iPro
- 38 CamExpert Memory Errors when Loading Camera Configuration Files
- 38 Overview of Sapera Acquisition Parameter Files (*.ccf or *.cca/*.cvi)
- 39 Camera Interfacing Check List
- 40 Linescan Example: Interfacing the Dalsa Piranha2 Linescan Camera
- 40 CamExpert Interfacing Outline
- 41 Step 1: Piranha2 in Free Run Exposure Mode
- 41 File Selection & Grab Test
- 43 Overview of Basic Timing Parameters
- 45 Step 2: Piranha2 in External Exposure Mode
- 45 CCF File Selection
- 46 Advanced Control Parameters
- 48 Step 3: Piranha2 with Shaft Encoder Line Sync
- 48 Shaft Encoder Line Sync Setup
- 50 Shaft Encoder with Fixed Frame Buffer Setup
- 52 Shaft Encoder with Variable Frame Buffer Setup
- 54 Using the Flat Field Correction Tool
- 54 X64-CL iPro Flat Field Support
- 54 Flat Field Correction Calibration Procedure
- 56 Using Flat Field Correction
- 56 Using the Bayer Filter Tool
- 56 Bayer Filter White Balance Calibration Procedure
- 57 Using the Bayer Filter
- 59 Grab Demo Overview
- 59 Using the Grab Demo
- 60 Flat-Field Demo Overview
- 60 Using the Flat Field Demo
- 61 X64-CL iPro Medium Block Diagram
- 62 X64-CL iPro Dual Base Block Diagram
- 63 X64-CL iPro\Lite Block Diagram
- 64 X64-CL iPro Acquisition Timing
- 65 Line Trigger Source Selection for Linescan Applications
- 65 CORACQ_PRM_EXT_LINE_TRIGGER_SOURCE – Parameter Values Specific to the X64-CL iPro series
- 67 Shaft Encoder Interface Timing
- 68 Virtual Frame Trigger for Linescan Cameras
- 69 Synchronization Signals for a Virtual Frame of 10 Lines.
- 70 Acquisition Methods
- 71 X64-CL iPro LUT availability
- 72 X64-CL iPro\Lite LUT availability
- 73 Trigger-to-Image Reliability
- 73 Supported Events and Transfer Methods
- 75 Trigger Signal Validity
- 75 Supported Transfer Cycling Methods
- 75 X64-CL iPro Sapera Parameters
- 76 Camera Related Parameters
- 81 VIC Related Parameters
- 85 ACQ Related Parameters
- 86 X64-CL iPro Memory Error with Area Scan Frame Buffer Allocation
- 87 Servers and Resources
- 88 Transfer Resource Locations
- 89 X64-CL iPro Board Specifications
- 91 X64-CL iPro\Lite Board Specifications
- 92 Host System Requirements
- 93 EMI Certifications
- 94 Connector and Switch Locations
- 94 X64-CL iPro Board Series Layout Drawings
- 95 Connector Description List
- 96 Connector and Switch Specifications
- 96 X64-CL iPro End Bracket
- 96 X64-CL iPro\Lite End Bracket
- 97 Status LEDs Functional Description
- 98 J1: Camera Link Connector 1 (applies to X64-CL iPro and X64-CL iPro\Lite)
- 98 J2: Camera Link Connector 2 (on X64-CL iPro with Dual Base Configuration)
- 99 J2: Camera Link Connector 2 (on X64-CL iPro in Medium Configuration)
- 100 Camera Link Camera Control Signal Overview
- 101 J4: External Signals Connector
- 101 J4 Signal Descriptions
- 104 X64-CL iPro: External Signals Connector Bracket Assembly
- 104 External Signals Connector Bracket Assembly Drawing
- 105 External Signals Connector Bracket Assembly Signal Description
- 105 Connecting a TTL Shaft Encoder Signal to the LVDS/RS422 Input
- 107 External Trigger TTL Input Electrical Specification
- 107 Strobe TTL Output Electrical Specification
- 108 J7: Board Sync
- 108 J8: Power to Camera Voltage Selector
- 109 J9: PC Power to Camera Interface
- 109 J11: Start Mode
- 109 J3, J12: Reserved
- 110 Brief Description of Standards RS-232, RS-422, & RS-644 (LVDS)
- 111 Camera Link Overview
- 111 Rights and Trademarks
- 112 Data Port Summary
- 112 Camera Signal Summary
- 112 Video Data
- 112 Camera Controls
- 113 Communication
- 113 Camera Link Cables
- 115 X-I/O Module Overview
- 116 X-I/O Module Connector List & Locations
- 116 X-I/O Module Installation
- 117 Board Installation
- 117 X64-CL iPro and X-I/O Driver Update
- 117 X-I/O Module External Connections to the DB37
- 118 DB37 Pinout Description
- 119 Outputs in NPN Mode: Electrical Details
- 120 Outputs in PNP Mode: Electrical Details
- 121 Opto-coupled Input: Electrical Details
- 121 TTL Input Electrical Details
- 122 X-I/O Module Sapera Interface
- 122 Configuring User Defined Power-up I/O States
- 123 Using Sapera LT General I/O Demo
- 126 Sapera LT General I/O Demo Code Samples
- 126 Main I/O Demo code
- 127 Function CreateObjects()
- 128 Output Dialog: CGioOutputDlg class (see Sapera Gui class)
- 128 Input Dialog: CGioInputDlg class. (see Sapera Gui class)
- 129 I/O Event Handling
- 131 Sales Information
- 132 Technical Support